Event-driven architecture (EDA) in Go involves designing applications as sets of loosely coupled, independent components that communicate with each other by exchanging events.
package mainimport ("fmt""log""github.com/aws/aws-lambda-go/events""github.com/aws/aws-lambda-go/lambda")func handleRequest(ctx context.Context, snsEvent events.SNSEvent) error {for _, record := range snsEvent.Records {snsRecord := record.SNSfmt.Printf("[%s %s] Message: %s\n", snsRecord.Timestamp, snsRecord.SignatureVersion, snsRecord.Message)}return nil}func main() {log.Printf("Golang Lambda starting...")lambda.Start(handleRequest)}
EDA has become increasingly popular in recent years due to its scalability, resilience, and ease of development. One of the key historical developments that led to the rise of EDA is the advent of cloud computing, which provides the infrastructure and services needed to implement EDA-based applications at scale.
In this article, we will explore the benefits of EDA, discuss best practices for implementing EDA-based applications in Go, and provide examples of how EDA is being used in real-world applications.
Exploring Event-Driven Architecture in Golang Applications
Event-driven architecture (EDA) has become increasingly popular in recent years due to its scalability, resilience, and ease of development. In this article, we will explore three key aspects of EDA in Golang applications:
- Decoupling: EDA decouples the components of an application, making it easier to scale and maintain.
- Concurrency: EDA allows applications to handle multiple events concurrently, improving performance and scalability.
- Resilience: EDA makes applications more resilient to failure by providing mechanisms for handling errors and recovering from failures.
These three aspects of EDA are essential for building scalable, resilient, and maintainable applications in Golang. By understanding and applying these concepts, developers can create applications that are well-suited for the demands of modern software development.
Decoupling
In the context of event-driven architecture (EDA), decoupling refers to the separation of an application’s components into independent units that communicate with each other through events. This approach offers several advantages, including:
- Scalability: Decoupled components can be scaled independently, making it easier to adjust the application’s capacity to meet changing demands.
- Maintainability: Decoupled components are easier to maintain and update, as changes to one component do not affect the other components.
- Resilience: Decoupled components make the application more resilient to failures, as a failure in one component does not necessarily affect the other components.
In Golang, decoupling can be achieved through the use of channels, message queues, and other mechanisms for sending and receiving events. By decoupling the components of an application, developers can create applications that are more scalable, maintainable, and resilient.
Concurrency
In event-driven architecture (EDA), concurrency refers to the ability of an application to handle multiple events simultaneously. This is in contrast to traditional request-response architectures, which handle requests one at a time. Concurrency is essential for building scalable and high-performance applications, as it allows the application to process more events in a shorter amount of time.
In Golang, concurrency can be achieved through the use of goroutines and channels. Goroutines are lightweight threads that can be used to execute code concurrently. Channels are used to communicate between goroutines and to pass data between them. By using goroutines and channels, developers can create applications that can handle multiple events concurrently, improving performance and scalability.
For example, consider an application that processes orders. In a traditional request-response architecture, each order would be processed one at a time. This could lead to performance problems if the application is receiving a large number of orders. In an EDA-based application, each order would be processed concurrently, using a goroutine to handle each order. This would allow the application to process orders much more quickly and efficiently.
Concurrency is an essential aspect of EDA and is critical for building scalable and high-performance applications in Golang. By understanding and applying the principles of concurrency, developers can create applications that can handle the demands of modern software development.
Resilience
In the context of event-driven architecture (EDA), resilience refers to the ability of an application to withstand and recover from failures. This is essential for building reliable and fault-tolerant applications, as failures are inevitable in any distributed system.
- Error handling: EDA provides mechanisms for handling errors in a graceful manner. For example, if an event cannot be processed successfully, it can be logged and retried later.
- Fault tolerance: EDA makes applications more fault-tolerant by isolating components from each other. This means that a failure in one component does not necessarily affect the other components.
- Recovery: EDA provides mechanisms for recovering from failures. For example, if a component fails, it can be restarted automatically.
- Scalability: EDA makes applications more scalable by allowing them to handle increased load without compromising reliability. This is because EDA applications can be easily scaled up or down by adding or removing components.
By providing mechanisms for handling errors, recovering from failures, and scaling up or down, EDA makes applications more resilient to failure. This is essential for building reliable and fault-tolerant applications in Golang.
FAQs on Event-Driven Architecture in Golang Applications
This section addresses frequently asked questions about event-driven architecture (EDA) in Golang applications.
Question 1: What are the benefits of using EDA in Golang applications?
EDA offers several benefits for Golang applications, including improved scalability, performance, resilience, and maintainability.
Question 2: How can I implement EDA in my Golang applications?
There are several ways to implement EDA in Golang applications. One common approach is to use channels and goroutines to decouple the components of the application and communicate between them using events.
Question 3: How can I ensure that my EDA applications are scalable?
To ensure scalability, it is important to design your EDA applications with loose coupling and independent components. This allows you to scale the application by adding or removing components as needed.
Question 4: How can I make my EDA applications more resilient?
To improve resilience, consider implementing mechanisms for handling errors, recovering from failures, and isolating components from each other. This will help ensure that your applications can withstand and recover from failures.
Question 5: What are some real-world examples of EDA in Golang applications?
EDA is used in a variety of real-world applications, including microservices architectures, data pipelines, and serverless applications.
Summary: EDA is a powerful architectural pattern that can be used to build scalable, resilient, and maintainable Golang applications. By understanding the benefits and best practices of EDA, developers can create applications that meet the demands of modern software development.
Transition to the next article section: In the next section, we will discuss the challenges of implementing EDA in Golang applications and explore some of the tools and frameworks that can help you overcome these challenges.
Tips for Exploring Event-Driven Architecture in Golang Applications
Event-driven architecture (EDA) is a powerful architectural pattern that can be used to build scalable, resilient, and maintainable Golang applications. Here are a few tips to help you get started with EDA:
Tip 1: Understand the benefits of EDA
Before you start implementing EDA, it is important to understand the benefits that it can offer. EDA can help you to improve the scalability, performance, resilience, and maintainability of your applications.
Tip 2: Choose the right tools and frameworks
There are a number of tools and frameworks available to help you implement EDA in Golang. Some of the most popular options include Apache Kafka, NATS, and RabbitMQ. Consider the specific requirements of your application when choosing a tool or framework.
Tip 3: Design your application with loose coupling
Loose coupling is a key principle of EDA. This means that the components of your application should be independent and loosely coupled. This will make your application more scalable and resilient.
Tip 4: Implement error handling and recovery mechanisms
Errors are inevitable in any distributed system. It is important to implement error handling and recovery mechanisms to ensure that your EDA application can withstand and recover from failures.
Tip 5: Monitor your application
Monitoring is essential for any production application. This is especially true for EDA applications, as they can be complex and difficult to debug. Use a monitoring tool to track the performance and health of your application.
Summary: EDA is a powerful architectural pattern that can be used to build scalable, resilient, and maintainable Golang applications. By following these tips, you can get started with EDA and start to reap the benefits that it can offer.
Conclusion
In this article, we have explored the benefits, best practices, and challenges of implementing event-driven architecture (EDA) in Golang applications.
EDA is a powerful architectural pattern that can be used to build scalable, resilient, and maintainable applications. By understanding the concepts of EDA and applying the best practices discussed in this article, developers can create applications that meet the demands of modern software development.
As EDA continues to evolve, we can expect to see even more innovative and efficient ways to implement this architectural pattern in Golang applications.
Youtube Video:
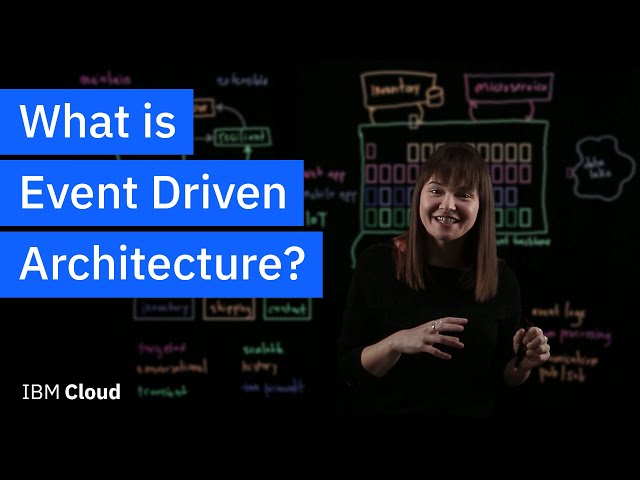