Profiling and Benchmarking in Golang: Enhancing Performance
func BenchmarkPrintVariable(b *testing.B) { for n := 0; n < b.N; n++ { fmt.Println(variable) } }
Profiling and Benchmarking in Golang refers to techniques used to analyze and measure the performance of Go programs. By identifying bottlenecks and inefficiencies, developers can optimize code for improved speed and resource utilization. This practice is crucial for building scalable and efficient applications.
Profiling and Benchmarking have been instrumental in performance optimization since the early days of computing. The advent of modern profiling tools and benchmarking frameworks has further simplified and enhanced these techniques, making them essential for any serious Golang developer seeking to deliver high-performance applications.
Profiling and Benchmarking in Golang
Profiling and Benchmarking are essential aspects of performance optimization in Golang. They provide valuable insights into the runtime behavior of programs, enabling developers to identify bottlenecks and inefficiencies.
- CPU Profiling: Analyze CPU usage and identify hotspots.
- Memory Profiling: Track memory allocation and detect leaks.
- Concurrency Profiling: Optimize goroutine scheduling and synchronization.
- Block Profiling: Identify blocking operations and improve responsiveness.
- Trace Analysis: Generate detailed execution traces for performance bottlenecks.
- Code Coverage: Measure the extent of code execution during tests.
- Performance Benchmarks: Establish performance baselines and compare optimizations.
- Load Testing: Simulate real-world usage patterns and assess scalability.
- Continuous Profiling: Integrate profiling into development pipelines for ongoing performance monitoring.
These aspects provide a comprehensive approach to performance optimization, covering various dimensions of program execution. By understanding and addressing these aspects, developers can significantly improve the performance, scalability, and efficiency of their Go applications.
CPU Profiling
CPU profiling is a critical aspect of Profiling and Benchmarking in Golang, providing deep insights into the runtime behavior of Go programs. By analyzing CPU usage and identifying hotspots, developers can pinpoint areas of code that consume excessive CPU resources, leading to performance bottlenecks and inefficiencies.
Real-life examples of CPU profiling in Profiling and Benchmarking in Golang include identifying performance issues in web servers, optimizing database queries, and improving the efficiency of machine learning algorithms. By identifying hotspots in these scenarios, developers can optimize code to reduce CPU usage, improve response times, and enhance overall performance.
The practical significance of understanding CPU profiling lies in its ability to empower developers with actionable insights to improve code quality. By identifying and addressing CPU hotspots, developers can create more efficient and scalable Go applications, ensuring optimal performance under varying workloads.
Memory Profiling
Memory profiling is a critical component of Profiling and Benchmarking in Golang, enabling developers to track memory allocation and detect leaks, which are major sources of performance degradation and instability in Go applications.
Real-life examples of memory profiling in Profiling and Benchmarking in Golang include identifying memory leaks in long-running web services, optimizing memory usage in data processing pipelines, and improving the performance of concurrent applications. By detecting and addressing memory leaks, developers can reduce memory consumption, improve application stability, and enhance overall performance.
The practical significance of understanding memory profiling lies in its ability to empower developers with actionable insights to improve code quality. By identifying and fixing memory leaks, developers can create more efficient and robust Go applications, ensuring optimal memory utilization and preventing performance issues caused by excessive memory usage.
Concurrency Profiling
Concurrency profiling is a critical component of Profiling and Benchmarking in Golang, enabling developers to optimize goroutine scheduling and synchronization for improved performance and scalability. Goroutines, lightweight threads in Go, are a fundamental aspect of concurrency, allowing for efficient execution of multiple tasks simultaneously.
Real-life examples of concurrency profiling in Profiling and Benchmarking in Golang include optimizing web servers for high concurrency, improving the performance of data processing pipelines, and enhancing the scalability of distributed systems. By identifying and addressing concurrency issues, developers can reduce contention, improve resource utilization, and enhance overall application performance.
The practical significance of understanding concurrency profiling lies in its ability to empower developers with actionable insights to improve code quality and performance. By identifying and fixing concurrency issues, developers can create more efficient and scalable Go applications, ensuring optimal performance under varying workloads and complex concurrency scenarios.
Block Profiling
Block profiling is a critical component of Profiling and Benchmarking in Golang, enabling developers to identify blocking operations that can significantly impact application performance and responsiveness. Blocking operations occur when a goroutine is paused while waiting for a resource or operation to complete, leading to underutilization of CPU and other resources.
Real-life examples of block profiling in Profiling and Benchmarking in Golang include optimizing web servers to reduce blocking I/O operations, improving the performance of database queries by identifying blocking locks, and enhancing the scalability of distributed systems by eliminating blocking communication patterns. By identifying and addressing blocking operations, developers can improve application throughput, reduce latency, and enhance overall responsiveness.
The practical significance of understanding block profiling lies in its ability to empower developers with actionable insights to improve code quality and performance. By identifying and fixing blocking operations, developers can create more efficient and responsive Go applications, ensuring optimal performance under varying workloads and complex concurrency scenarios.
Trace Analysis
Trace analysis is a critical component of Profiling and Benchmarking in Golang, enabling developers to generate detailed execution traces that provide insights into the runtime behavior of their applications. These traces can be analyzed to identify performance bottlenecks, understand the flow of execution, and pinpoint the root causes of performance issues.
Real-life examples of trace analysis in Profiling and Benchmarking in Golang include identifying performance issues in distributed systems, optimizing the performance of web applications, and debugging complex concurrency issues. By analyzing execution traces, developers can gain a deep understanding of how their applications behave under load, leading to targeted optimizations and performance improvements.
The practical significance of understanding trace analysis lies in its ability to empower developers with actionable insights to improve code quality and performance. By identifying and addressing performance bottlenecks through trace analysis, developers can create more efficient and scalable Go applications, ensuring optimal performance under varying workloads and complex scenarios.
Code Coverage
Code coverage is a critical component of Profiling and Benchmarking in Golang, providing insights into the extent of code execution during tests. By measuring the percentage of code that is executed during test runs, developers can assess the effectiveness of their test suites and identify areas of code that are not covered by tests.
Real-life examples of code coverage within Profiling and Benchmarking in Golang include ensuring that critical code paths are adequately tested, identifying untested corner cases, and improving test coverage for complex and evolving codebases. By analyzing code coverage reports, developers can prioritize testing efforts, improve code quality, and reduce the risk of undetected bugs.
The practical significance of understanding code coverage lies in its ability to empower developers with actionable insights to improve the reliability and maintainability of their Go applications. By identifying and addressing areas with low code coverage, developers can create more robust and comprehensive test suites, leading to increased confidence in the quality and correctness of their code.
Performance Benchmarks
Performance benchmarks are a critical component of Profiling and Benchmarking in Golang, providing essential insights into the performance characteristics of Go applications. By establishing performance baselines and comparing optimizations, developers can quantify the impact of changes to their code, ensuring that optimizations deliver tangible performance improvements.
Real-life examples of performance benchmarks within Profiling and Benchmarking in Golang include comparing the performance of different algorithms for a specific task, evaluating the impact of code optimizations on application latency, and assessing the scalability of applications under varying loads. By establishing performance baselines, developers can track progress over time and identify areas for further optimization.
The practical significance of understanding performance benchmarks lies in their ability to empower developers with data-driven insights to make informed decisions about code optimizations. By comparing optimizations and analyzing performance results, developers can prioritize performance improvements, identify bottlenecks, and ensure that their applications meet performance requirements. Performance benchmarks are essential for continuous performance monitoring and improvement, enabling developers to deliver high-quality, performant Go applications.
Load Testing
Load testing plays a critical role in Profiling and Benchmarking in Golang by simulating real-world usage patterns and assessing the scalability of applications. It helps identify performance bottlenecks and ensures that applications can handle expected loads without performance degradation.
- Concurrency and Load Simulation: Simulate multiple concurrent users accessing the application to evaluate its ability to handle high concurrency and load.
- Scalability Assessment: Determine the maximum capacity of the application by gradually increasing the load and monitoring performance metrics.
- Performance Under Load: Identify performance bottlenecks and areas for optimization by analyzing metrics such as response times, throughput, and resource utilization.
- Stress Testing: Subject the application to extreme load conditions beyond normal usage patterns to assess its resilience and identify potential failure points.
By incorporating load testing into Profiling and Benchmarking in Golang, developers can ensure that their applications are scalable, reliable, and capable of handling real-world usage patterns. This leads to improved performance, increased user satisfaction, and reduced risk of performance issues in production environments.
Continuous Profiling
Continuous Profiling is a critical component of Profiling and Benchmarking in Golang as it enables developers to integrate profiling into their development pipelines, ensuring ongoing performance monitoring and optimization. By continuously collecting performance data throughout the development lifecycle, developers can identify and address performance issues early on, preventing them from propagating to production environments.
Real-life examples of Continuous Profiling in Profiling and Benchmarking in Golang include:
- Monitoring performance metrics during development to identify potential bottlenecks and optimize code.
- Integrating performance profiling into automated testing pipelines to ensure that performance requirements are met.
- Regularly profiling production systems to identify performance degradation and proactively address issues.
The practical significance of understanding Continuous Profiling lies in its ability to empower developers with actionable insights to improve code quality and performance continuously. By integrating profiling into development pipelines, developers can establish performance baselines, track performance over time, and identify areas for optimization. This leads to improved application performance, reduced development time, and increased confidence in the reliability of Go applications.
Frequently Asked Questions about Profiling and Benchmarking in Golang
This FAQ section addresses common questions and clarifies important aspects of Profiling and Benchmarking in Golang, helping you gain a deeper understanding of this topic.
Question 1: What is the primary objective of Profiling and Benchmarking in Golang?
Profiling and Benchmarking in Golang provide valuable techniques to analyze and improve the performance of Go programs, ensuring they operate efficiently and meet performance requirements.
Question 2: What specific benefits can I expect from Profiling and Benchmarking my Go code?
Profiling and Benchmarking offer numerous benefits, including identifying performance bottlenecks, optimizing resource utilization, enhancing scalability, and ensuring code quality.
Question 3: What are the key aspects of Go program performance that Profiling and Benchmarking can address?
Profiling and Benchmarking cover various aspects of Go performance, such as CPU usage, memory allocation, concurrency, blocking operations, and overall execution efficiency.
Question 4: How can I integrate Profiling and Benchmarking into my Go development workflow?
Profiling and Benchmarking can be integrated into your workflow using built-in Go tools, third-party profiling frameworks, and continuous profiling techniques.
Question 5: What are some real-world examples of how Profiling and Benchmarking have improved Go applications?
Real-world examples include optimizing web servers for high concurrency, improving the performance of data processing pipelines, and enhancing the scalability of distributed systems.
Question 6: How can Profiling and Benchmarking help me deliver high-quality and performant Go applications?
By understanding and applying Profiling and Benchmarking techniques, you can identify and resolve performance issues, leading to more efficient, reliable, and scalable Go applications.
In summary, Profiling and Benchmarking are essential practices for performance optimization in Golang. This FAQ section has provided insights into their significance, benefits, and practical applications. In the following section, we will explore specific techniques and tools for Profiling and Benchmarking in Golang, empowering you to optimize the performance of your Go applications.
Tips for Profiling and Benchmarking in Golang
This section provides actionable tips to enhance your Profiling and Benchmarking practices in Golang, enabling you to optimize the performance of your applications effectively.
1. Identify Performance Bottlenecks with Profiling
Use profiling tools to identify performance bottlenecks and pinpoint areas of your code that require optimization. This can help you focus your efforts on improving the most critical aspects of your application.
2. Establish Performance Benchmarks
Set performance benchmarks to measure the performance of your application before and after optimizations. This will provide a baseline for comparison and help you quantify the impact of your changes.
3. Perform Load Testing
Simulate real-world usage patterns through load testing to assess the scalability and performance of your application under load. This helps you identify potential issues and ensure your application can handle expected traffic.
4. Integrate Continuous Profiling
Incorporate profiling into your development pipeline to continuously monitor the performance of your application. This allows you to identify performance issues early on and prevent them from propagating to production.
5. Optimize CPU Usage
Analyze CPU usage profiles to identify areas where your code consumes excessive CPU resources. Optimize these areas by reducing computational complexity, parallelizing tasks, or improving algorithm efficiency.
6. Manage Memory Effectively
Use memory profiles to track memory allocation and detect memory leaks. Improve memory management by optimizing data structures, avoiding unnecessary allocations, and properly releasing resources.
7. Concurrency Optimization
Analyze concurrency profiles to identify goroutine scheduling and synchronization issues. Optimize concurrency by reducing contention, balancing goroutine workloads, and using appropriate synchronization primitives.
8. Trace Execution Flow
Generate execution traces to visualize the flow of execution and identify potential bottlenecks. Analyze traces to understand the sequence of events and pinpoint areas for optimization.
By following these tips, you can effectively Profile and Benchmark your Go applications, leading to improved performance, scalability, and resource efficiency.
In the next section, we will explore specific tools and techniques for Profiling and Benchmarking in Golang, empowering you to apply these tips in practice and achieve optimal performance for your applications.
Conclusion
In this article, we have explored the significance and practical applications of Profiling and Benchmarking in Golang for enhancing application performance. Through detailed explanations and actionable tips, we have shed light on key aspects such as identifying performance bottlenecks, establishing benchmarks, optimizing CPU and memory usage, and leveraging concurrency effectively.
Profiling and Benchmarking are essential practices for any Go developer who seeks to deliver high-quality, performant applications. By embracing these techniques, developers can gain deep insights into the runtime behavior of their code, identify areas for improvement, and ensure that their applications meet performance requirements. Continuously Profiling and Benchmarking throughout the development lifecycle empowers developers to proactively address performance issues and maintain optimal application performance.
In summary, Profiling and Benchmarking are indispensable tools for performance optimization in Golang. By understanding and applying the techniques discussed in this article, developers can create scalable, efficient, and responsive Go applications that meet the demands of modern software systems.
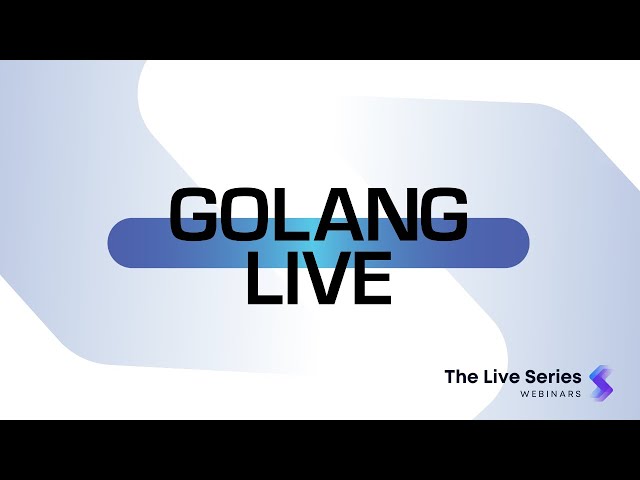