In the realm of web development, crafting robust REST APIs is paramount. Among the myriad frameworks available, Echo Framework in Go stands out as a formidable choice. This article delves into the advanced techniques employed in creating REST APIs using Echo Framework, empowering developers to harness its full potential.
package mainimport ("net/http""github.com/labstack/echo/v4")func main() {e := echo.New()e.GET("/", func(c echo.Context) error {return c.String(http.StatusOK, "Hello, World!")})e.Logger.Fatal(e.Start(":1323"))}
Echo Framework is renowned for its elegance, performance, and extensibility. Its focus on simplicity and ease of use makes it a favorite among developers seeking to expedite their API development process. Historically, Echo Framework emerged as a response to the need for a lightweight and efficient alternative to existing frameworks, quickly gaining popularity due to its exceptional features.
Throughout this article, we will explore advanced techniques for working with Echo Framework, including:
- Customizing HTTP responses
- Middleware for request and response processing
- Error handling and recovery
- Testing and debugging
By delving into these techniques, developers can unlock the full potential of Echo Framework and create robust, scalable, and maintainable REST APIs.
Creating REST APIs with Echo Framework in Golang
In the realm of web development, REST APIs are ubiquitous, and Echo Framework has emerged as a formidable tool for crafting robust and efficient APIs in Go. This article delves into three key aspects of advanced REST API development with Echo Framework:
- Middleware: Intercepts and processes requests and responses, enabling tasks like authentication, logging, and rate limiting.
- Error Handling: Provides a structured approach to handling errors, ensuring a consistent and user-friendly API experience.
- Testing: Facilitates the creation of comprehensive tests for REST APIs, ensuring their reliability and correctness.
These aspects are interconnected and essential for building scalable, maintainable, and user-friendly REST APIs. Middleware allows developers to enhance the functionality of their APIs without modifying the core API code. Error handling provides a consistent and informative way to handle errors, improving the developer and user experience. Thorough testing ensures the reliability and correctness of APIs, reducing the likelihood of unexpected behavior in production.
Overall, mastering these advanced techniques will empower developers to create robust, efficient, and well-tested REST APIs using Echo Framework in Go.
Middleware
Middleware plays a pivotal role in creating robust and efficient REST APIs with Echo Framework in Golang. It acts as an intermediary between the client and the API, intercepting and processing requests and responses. This capability empowers developers to implement essential tasks such as authentication, logging, and rate limiting without modifying the core API code.
Consider authentication as an example. Middleware can be used to verify the identity of users attempting to access API resources. This ensures that only authorized users have access to sensitive data or functionality. Middleware can also be used to log API requests and responses, providing valuable insights into API usage and performance. Additionally, middleware can be employed to implement rate limiting, preventing excessive API usage and protecting against denial-of-service attacks.
In summary, middleware is a powerful tool that enhances the functionality and security of REST APIs. By leveraging middleware, developers can implement essential tasks like authentication, logging, and rate limiting, resulting in more robust, efficient, and secure APIs.
Error Handling
In the context of creating REST APIs with Echo Framework in Go, error handling is of paramount importance. A well-structured error handling mechanism ensures that errors are handled consistently and in a user-friendly manner, enhancing the overall developer and user experience.
-
Error Classification and Categorization
Echo Framework provides a comprehensive approach to error classification and categorization. Errors are classified into different types, such as client errors, server errors, and internal errors. This categorization helps developers identify the root cause of errors quickly and efficiently.
-
Custom Error Messages
Echo Framework empowers developers to define custom error messages for different error types. This customization allows developers to provide user-friendly error messages that are tailored to the specific context of their API. This enhances the developer experience and simplifies debugging.
-
Error Middleware
Error middleware can be implemented to handle errors in a centralized and structured manner. This middleware can log errors, return appropriate HTTP status codes, and provide user-friendly error messages. Error middleware simplifies error handling and ensures consistency across the entire API.
-
Testing and Debugging
Echo Framework provides excellent support for testing and debugging error handling mechanisms. Developers can write comprehensive tests to verify that errors are handled as expected. Additionally, Echo Framework provides debugging tools that help developers identify and resolve errors quickly.
By leveraging the advanced error handling capabilities of Echo Framework, developers can create REST APIs that are robust, reliable, and user-friendly. Effective error handling enhances the overall quality and usability of APIs, ensuring a positive experience for both developers and end-users.
Testing
In the realm of creating REST APIs with Echo Framework in Go, testing plays a pivotal role in ensuring the reliability and correctness of APIs. Comprehensive tests provide developers with confidence that their APIs function as intended, handling various scenarios and edge cases effectively.
Echo Framework provides robust testing support, enabling developers to write unit tests, integration tests, and end-to-end tests. Unit tests focus on individual components or functions, while integration tests verify the interactions between different components. End-to-end tests simulate real-world usage scenarios, ensuring that the API behaves as expected in its entirety.
The importance of testing cannot be overstated. By investing in comprehensive testing, developers can identify and resolve issues early in the development cycle, reducing the likelihood of defects in production. Well-tested APIs are more stable, reliable, and less prone to unexpected behavior, enhancing the overall user experience.
Moreover, testing facilitates continuous integration and continuous delivery (CI/CD) pipelines, enabling developers to automate the testing process and quickly deliver new features and bug fixes. Automated testing ensures that code changes do not inadvertently introduce errors, maintaining the quality and reliability of the API over time.
In conclusion, testing is an indispensable aspect of creating REST APIs with Echo Framework in Go. By leveraging the testing capabilities of Echo Framework, developers can create robust, reliable, and correct APIs that meet the demands of modern web applications.
FAQs on Creating REST APIs with Echo Framework in Golang
This section addresses commonly asked questions and misconceptions regarding the creation of REST APIs using Echo Framework in Golang.
Question 1: What are the key benefits of using Echo Framework for REST API development?
Answer: Echo Framework offers several advantages, including its simplicity, performance, extensibility, and focus on ease of use. It empowers developers to create robust and efficient APIs with minimal effort.
Question 2: How does middleware enhance the capabilities of REST APIs built with Echo Framework?
Answer: Middleware acts as an intermediary, intercepting and processing requests and responses. It enables essential tasks such as authentication, logging, and rate limiting without modifying the core API code, enhancing functionality and security.
Question 3: What is the significance of error handling in REST API development using Echo Framework?
Answer: Error handling is crucial for ensuring the reliability and user-friendliness of REST APIs. Echo Framework provides a structured approach to error classification, categorization, and handling, allowing developers to provide informative error messages and handle errors consistently.
Question 4: How does testing contribute to the quality of REST APIs built with Echo Framework?
Answer: Testing is essential for verifying the correctness and reliability of REST APIs. Echo Framework supports unit testing, integration testing, and end-to-end testing, enabling developers to identify and resolve issues early in the development cycle.
Question 5: What are some best practices for creating REST APIs with Echo Framework?
Answer: Best practices include utilizing middleware effectively, implementing robust error handling mechanisms, writing comprehensive tests, adhering to RESTful design principles, and leveraging Echo Framework’s features to enhance performance and security.
Summary: Creating REST APIs with Echo Framework in Golang requires a combination of technical expertise, attention to detail, and a focus on best practices. By mastering the techniques discussed in this article, developers can create robust, scalable, and user-friendly APIs that meet the demands of modern web applications.
Transition to the next article section: In the next section, we will delve into the practical implementation of these advanced techniques, providing code examples and step-by-step guidance to help you create effective REST APIs using Echo Framework in Golang.
Tips for Creating REST APIs with Echo Framework in Golang
To enhance your API development process, consider the following tips:
Tip 1: Leverage Middleware Effectively
Middleware provides a powerful mechanism to extend the functionality of your APIs without modifying the core code. Utilize middleware to implement essential tasks such as authentication, authorization, logging, and rate limiting.
Tip 2: Implement Robust Error Handling
Handle errors gracefully and consistently by implementing a structured error handling mechanism. Echo Framework provides comprehensive support for error classification, categorization, and handling, enabling you to provide informative error messages and enhance the user experience.
Tip 3: Prioritize Testing
Ensure the reliability and correctness of your APIs through comprehensive testing. Write unit tests, integration tests, and end-to-end tests to verify the functionality and behavior of your APIs under various scenarios.
Tip 4: Adhere to RESTful Design Principles
Follow RESTful design principles to create well-structured and consistent APIs. Utilize appropriate HTTP methods, status codes, and resource representations to enhance the usability and interoperability of your APIs.
Tip 5: Leverage Echo Framework’s Features
Explore and leverage Echo Framework’s rich set of features to enhance the performance, security, and maintainability of your APIs. Utilize features such as routing, validation, and dependency injection to streamline your development process.
Conclusion
In this article, we have explored advanced techniques for creating REST APIs with Echo Framework in Golang, empowering you to develop robust, scalable, and user-friendly APIs.
We have emphasized the significance of middleware, error handling, testing, and adherence to RESTful design principles. By leveraging these techniques and the capabilities of Echo Framework, you can craft APIs that meet the demands of modern web applications.
Youtube Video:
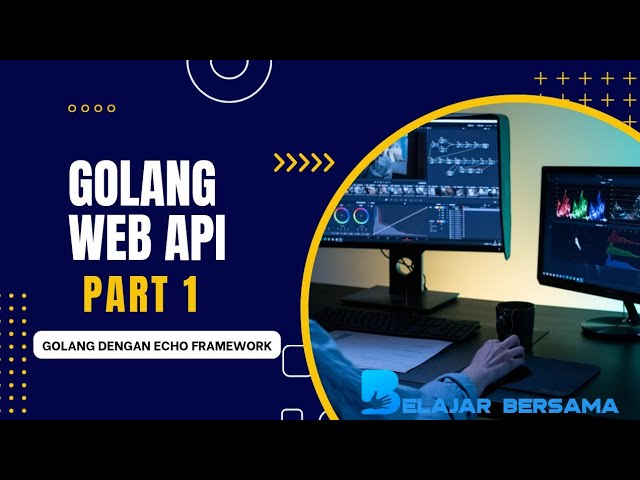