Implementing Clean Architecture in Golang Projects: Best Practices refers to the process of structuring a Golang application using the Clean Architecture pattern, which is a software design pattern that aims to achieve separation of concerns, testability, and maintainability.
type Service interface { CreateTask(ctx context.Context, task model.Task) error GetTask(ctx context.Context, id string) (model.Task, error) UpdateTask(ctx context.Context, id string, updatedTask model.Task) (model.Task, error) DeleteTask(ctx context.Context, id string) error }
The Clean Architecture pattern is particularly relevant for large-scale Golang projects, as it helps to manage complexity and reduce the risk of introducing bugs. Additionally, it has become increasingly popular due to the rise of microservices and serverless architectures, which have placed a greater emphasis on modularity and reusability.
In this article, we will discuss the key principles and best practices for implementing Clean Architecture in Golang projects. We will cover topics such as structuring your application, defining interfaces, and using dependency injection.
Implementing Clean Architecture in Golang Projects
Implementing Clean Architecture in Golang projects involves adhering to certain best practices to ensure effective software design and development. Two key aspects to consider are:
- Modularity: Breaking down the application into independent, cohesive modules that can be easily combined and reused.
- Dependency Injection: Passing dependencies to objects through their constructors or setters, rather than creating them internally, which enhances testability and loose coupling.
These aspects play a crucial role in implementing Clean Architecture in Golang projects. Modularity allows for better organization and maintenance of code, while dependency injection promotes flexibility and reduces the risk of introducing bugs. By following these best practices, developers can create Golang applications that are maintainable, extensible, and testable.
Modularity
In the context of Implementing Clean Architecture in Golang projects, modularity is a key principle that involves decomposing the application into a set of loosely coupled, independent modules. Each module should be cohesive, meaning that it performs a specific set of related tasks and has a well-defined interface. By breaking down the application into modules, it becomes easier to manage complexity, improve maintainability, and facilitate code reuse.
Modularity is particularly important in large-scale Golang projects, where the application may consist of numerous components and features. By organizing the code into modules, developers can more easily understand and manage the dependencies between different parts of the application. Additionally, modularity enables developers to isolate and test individual modules independently, which can significantly reduce the time and effort required for testing and debugging.
In practice, modularity can be achieved through a variety of techniques, such as using packages, interfaces, and dependency injection. By following best practices for modular design, developers can create Golang applications that are easier to maintain, extend, and test.
Dependency Injection
Dependency injection is a software design pattern that involves passing dependencies to objects through their constructors or setters, rather than creating them internally. This technique enhances testability and loose coupling, which are key principles of Clean Architecture.
In the context of Implementing Clean Architecture in Golang projects, dependency injection plays a crucial role in ensuring that the application is maintainable, extensible, and testable. By passing dependencies explicitly, developers can avoid creating tightly coupled code, where changes to one part of the application can have unintended consequences in other parts. This makes it easier to test individual components independently and to refactor the code without introducing bugs.
For example, consider a Golang application that uses a database to store and retrieve data. If the application creates the database connection internally, it becomes difficult to test the code that uses the database, as the test would need to also create and manage the database connection. By using dependency injection, the database connection can be passed to the code that uses it, making it easier to test the code in isolation.
Overall, dependency injection is a powerful technique that can significantly improve the quality of Golang applications. By following best practices for dependency injection, developers can create applications that are easier to maintain, extend, and test.
FAQs on Implementing Clean Architecture in Golang Projects
This section addresses frequently asked questions (FAQs) about implementing Clean Architecture in Golang projects, providing concise and informative answers to common concerns or misconceptions.
Question 1: What are the key benefits of using Clean Architecture in Golang projects?
Clean Architecture offers several key benefits, including improved modularity, maintainability, testability, and extensibility. It helps to organize code into distinct layers and components, making it easier to understand, modify, and test individual parts of the application.
Question 2: How can I effectively apply dependency injection in my Golang project?
To effectively apply dependency injection, consider using a dependency injection framework or library. This can help to simplify the process of passing dependencies to objects and ensure that they are properly resolved throughout the application.
Question 3: What are some best practices for structuring Golang applications using Clean Architecture?
Best practices include organizing code into well-defined layers, such as domain, application, and infrastructure layers. Each layer should have a clear and focused purpose, and dependencies should flow from higher layers to lower layers.
Question 4: How can I ensure that my Golang application adheres to Clean Architecture principles?
To ensure adherence to Clean Architecture principles, consider using tools or frameworks that support the pattern. Additionally, regular code reviews and adherence to best practices can help to maintain the integrity of the application’s architecture.
Question 5: What are some common challenges in implementing Clean Architecture in Golang projects?
Common challenges include managing the complexity of large-scale projects, ensuring consistency in code style and adherence to best practices, and addressing legacy code that may not conform to the Clean Architecture pattern.
Summary: Implementing Clean Architecture in Golang projects requires careful planning and adherence to best practices. By understanding the principles and benefits of Clean Architecture, developers can create applications that are maintainable, extensible, and testable.
Transition to the next article section: In the next section, we will delve deeper into the practical aspects of implementing Clean Architecture in Golang projects, providing detailed guidance and code examples.
Tips for Implementing Clean Architecture in Golang Projects
Implementing Clean Architecture in Golang projects requires careful planning and adherence to best practices. Here are a few tips to help you get started:
Tip 1: Start with a clear understanding of the Clean Architecture principles.
Before you start implementing Clean Architecture in your Golang project, it is important to have a clear understanding of the principles behind the pattern. This will help you to make informed decisions about how to structure your code and how to apply the principles to your specific project.
Tip 2: Use a layered architecture.
Clean Architecture is based on a layered architecture. This means that your code should be organized into distinct layers, such as a domain layer, an application layer, and an infrastructure layer. Each layer should have a clear and focused purpose, and dependencies should flow from higher layers to lower layers.
Tip 3: Use dependency injection.
Dependency injection is a technique that allows you to pass dependencies to objects through their constructors or setters. This helps to decouple your code and make it more testable. There are a number of dependency injection frameworks available for Golang, such as Wire and Gin.
Tip 4: Use interfaces to define your dependencies.
When you use dependency injection, it is important to use interfaces to define your dependencies. This will allow you to mock your dependencies during testing. Additionally, using interfaces will help you to keep your code loosely coupled.
Tip 5: Test your code regularly.
Testing is an important part of any software development process. When you are implementing Clean Architecture in your Golang project, it is important to test your code regularly. This will help you to ensure that your code is working as expected and that it is following the Clean Architecture principles.
Conclusion
In this article, we have explored the key principles and best practices for implementing Clean Architecture in Golang projects. We have discussed the benefits of using Clean Architecture, such as improved modularity, maintainability, testability, and extensibility. We have also provided tips on how to effectively apply Clean Architecture in your own projects.
By following the principles and best practices outlined in this article, you can create Golang applications that are maintainable, extensible, and testable. This will help you to build high-quality software that meets the needs of your users.
Youtube Video:
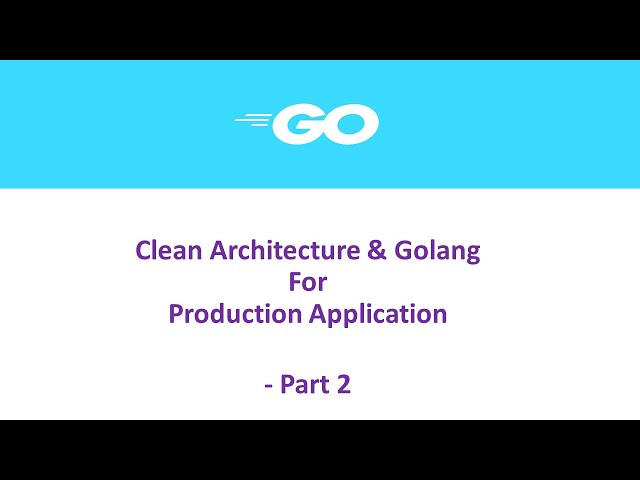