REST APIs, or Representational State Transfer APIs, are a type of web API that uses HTTP requests to create, read, update, and delete data. They are widely used in modern web development due to their flexibility and scalability. Fiber Framework is a popular Golang web framework that makes it easy to build REST APIs.
To get started with building REST APIs with Fiber Framework, you first need to install the framework using the following command:
go get -u github.com/gofiber/fiber/v2
Once you have installed Fiber Framework, you can create a new project and add the following code to your main.go file:
package mainimport ("github.com/gofiber/fiber/v2")func main() {app := fiber.New()app.Get("/", func(c *fiber.Ctx) error {return c.SendString("Hello, World!")})app.Listen(":3000")}
This code creates a new Fiber app and defines a route that responds to GET requests to the root URL (“/”) with the message “Hello, World!”. You can then run your app using the following command:
go run main.go
Once your app is running, you can open your browser and navigate to http://localhost:3000 to see the “Hello, World!” message.
This is just a simple example of how to build a REST API with Fiber Framework. For more information, please refer to the Fiber Framework documentation.
Building REST APIs with Fiber Framework in Golang
REST APIs are a popular choice for web development due to their flexibility and scalability. Fiber Framework is a popular Golang web framework that makes it easy to build REST APIs. In this article, we will discuss three key aspects of building REST APIs with Fiber Framework: installation, routing, and middleware.
-
Installation: Installing Fiber Framework is simple and straightforward. You can install it using the following command:
go get -u github.com/gofiber/fiber/v2
- Routing: Fiber Framework provides a powerful routing system that makes it easy to define routes for your API. You can define routes for different HTTP methods, such as GET, POST, PUT, and DELETE. For example, the following code defines a route that responds to GET requests to the root URL (“/”) with the message “Hello, World!”:
app.Get("/", func(c *fiber.Ctx) error {return c.SendString("Hello, World!")})
Middleware: Middleware is a powerful feature of Fiber Framework that allows you to add functionality to your API without modifying your routes. For example, you can use middleware to authenticate users, log requests, or compress responses.
These are just a few of the key aspects of building REST APIs with Fiber Framework. For more information, please refer to the Fiber Framework documentation.
Installation
The installation of Fiber Framework is a crucial step in building REST APIs with Fiber Framework in Golang. Without proper installation, you will not be able to use Fiber Framework to create your API. The installation process is simple and straightforward, as described in the provided command. Once Fiber Framework is installed, you can begin defining routes for your API. Routing is a fundamental aspect of building REST APIs, as it allows you to specify how your API will respond to different HTTP requests. Fiber Framework provides a powerful routing system that makes it easy to define routes for different HTTP methods and URLs. This allows you to create a flexible and scalable API that can handle a variety of requests.
In summary, the installation of Fiber Framework and the use of its routing system are essential components of building REST APIs with Fiber Framework in Golang. By understanding the importance of these components, you can create robust and efficient APIs that meet the needs of your application.
Middleware
Middleware is a crucial component of building REST APIs with Fiber Framework in Golang. It allows you to add functionality to your API without modifying your routes, making it easier to maintain and extend your API. For example, you can use middleware to:
- Authenticate users
- Log requests
- Compress responses
Middleware is a powerful tool that can help you improve the security, performance, and maintainability of your API. It is an essential component of building REST APIs with Fiber Framework in Golang.
Here is an example of how to use middleware to authenticate users:
app.Use(func(c *fiber.Ctx) error { // Get the authorization header from the request. authHeader := c.Get("Authorization") // Check if the authorization header is present. if authHeader == "" { // If the authorization header is not present, return an unauthorized error. return c.Status(401).SendString("Unauthorized") } // Parse the authorization header. token, err := ParseAuthorizationHeader(authHeader) if err != nil { // If the authorization header is invalid, return an unauthorized error. return c.Status(401).SendString("Unauthorized") } // Validate the token. user, err := ValidateToken(token) if err != nil { // If the token is invalid, return an unauthorized error. return c.Status(401).SendString("Unauthorized") } // Set the user on the context. c.Locals("user", user) // Continue to the next middleware or route. c.Next() })
This middleware will check if the authorization header is present and valid. If the authorization header is not present or invalid, the middleware will return an unauthorized error. If the authorization header is valid, the middleware will parse the token and validate it. If the token is valid, the middleware will set the user on the context and continue to the next middleware or route. Middleware is a powerful tool that can help you improve the security, performance, and maintainability of your API. It is an essential component of building REST APIs with Fiber Framework in Golang.
FAQs on Building REST APIs with Fiber Framework in Golang
This section addresses frequently asked questions (FAQs) to provide a comprehensive understanding of building REST APIs with Fiber Framework in Golang.
Question 1: What are the key benefits of using Fiber Framework for building REST APIs?
Fiber Framework offers several advantages, including high performance, ease of use, and a comprehensive feature set. Its optimized architecture enables fast and efficient handling of HTTP requests, while its intuitive syntax and extensive documentation simplify the development process.
Question 2: How does Fiber Framework handle routing in REST APIs?
Fiber Framework’s routing system provides a flexible and powerful mechanism for defining routes based on HTTP methods and URL patterns. It supports various HTTP verbs (GET, POST, PUT, DELETE, etc.) and allows for custom routing configurations to handle complex request scenarios.
Question 3: What is middleware, and how is it utilized in Fiber Framework?
Middleware in Fiber Framework serves as an essential tool for adding functionality to API routes. It enables developers to intercept and process requests before they reach the eigentlichen route handler. Middleware can be used for tasks such as authentication, logging, and error handling, enhancing the security and efficiency of the API.
Question 4: How does Fiber Framework facilitate data validation in REST APIs?
Fiber Framework provides built-in support for data validation, allowing developers to define validation rules for request parameters. This helps ensure that the API receives high-quality data, reducing the risk of errors and improving the overall reliability of the application.
Question 5: What are the best practices for maintaining REST APIs built with Fiber Framework?
To maintain the health and performance of REST APIs, it is crucial to follow best practices such as proper error handling, implementing security measures, monitoring API usage, and regularly updating the framework and its dependencies.
Question 6: Where can I find additional resources and support for building REST APIs with Fiber Framework?
The Fiber Framework community offers extensive documentation, tutorials, and examples. Developers can also engage in online forums and discussion groups to connect with other users, share knowledge, and seek assistance with specific challenges.
In summary, building REST APIs with Fiber Framework in Golang offers numerous advantages and involves key concepts such as routing, middleware, data validation, and maintenance. By leveraging the capabilities of Fiber Framework and adhering to best practices, developers can create robust, efficient, and scalable REST APIs.
Transition to the next article section:
Building REST APIs with Fiber Framework in Golang
This section delves into practical examples to illustrate the concepts and techniques involved in building REST APIs with Fiber Framework in Golang.
Example 1: Defining a Simple Route
This example demonstrates how to define a simple route that responds to GET requests to the root URL (“/”) with the message “Hello, World!”.
package mainimport ("github.com/gofiber/fiber/v2")func main() {app := fiber.New()app.Get("/", func(c fiber.Ctx) error {return c.SendString("Hello, World!")})app.Listen(":3000")}
Notes: This example showcases the basic structure of a Fiber application and the use of the Get() method to define a route.
Example 2: Using Middleware for Authentication
This example illustrates how to use middleware to authenticate users before they can access certain routes.
package mainimport ("github.com/gofiber/fiber/v2")func main() {app := fiber.New()app.Use(func(c
fiber.Ctx) error {// Authentication logic here})app.Get(“/protected”, func(c fiber.Ctx) error {return c.SendString(“Welcome, authenticated user!”)})app.Listen(“:3000”)}
Notes: This example demonstrates the use of middleware to add functionality to the API without modifying the routes themselves.
Example 3: Validating Request Data
This example shows how to validate request data using the built-in validation features of Fiber Framework.
package mainimport ("github.com/gofiber/fiber/v2")func main() {app := fiber.New()app.Post("/user", func(c
fiber.Ctx) error {// Validation logic here})app.Listen(“:3000”)}
Notes: This example showcases how to ensure that the API receives high-quality data by enforcing validation rules on request parameters.
Summary:These examples provide a practical understanding of the key concepts involved in building REST APIs with Fiber Framework in Golang. By leveraging the framework’s features and adhering to best practices, developers can create robust, efficient, and scalable APIs.
Transition to the article’s conclusion:In conclusion, Fiber Framework offers a comprehensive and developer-friendly solution for building REST APIs in Golang. Its high performance, ease of use, and extensive feature set make it an ideal choice for both beginners and experienced developers.
Conclusion
In this article, we have explored the fundamentals of building REST APIs with Fiber Framework in Golang. We discussed the installation process, routing mechanisms, and the use of middleware for enhancing API functionality. By leveraging the features of Fiber Framework and adhering to best practices, developers can create robust, efficient, and scalable REST APIs.
As the landscape of web development continues to evolve, Fiber Framework remains a compelling choice for building high-performance and user-friendly APIs. Its active community and extensive documentation provide ongoing support and resources for developers. We encourage readers to delve deeper into Fiber Framework’s capabilities and explore its potential for building innovative and effective web applications.
Youtube Video:
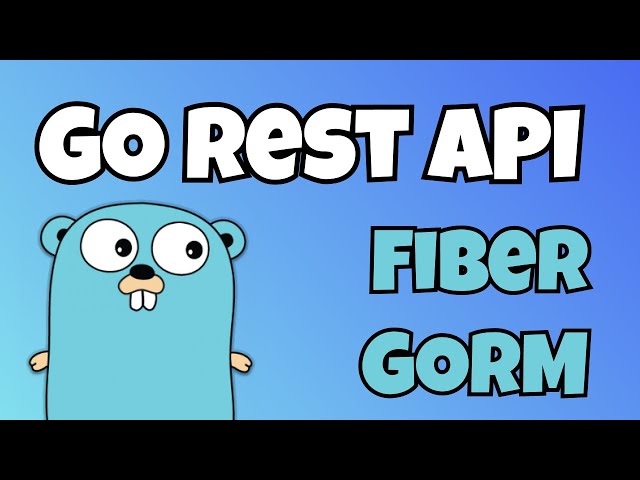