Cloud Database Management refers to the practice of managing and administering databases in the cloud, offering benefits such as scalability, flexibility, and cost-effectiveness. One popular cloud database solution is MongoDB Atlas, which provides a fully managed MongoDB database service on the cloud.
package mainimport ("context""fmt""log""go.mongodb.org/mongo-driver/mongo""go.mongodb.org/mongo-driver/mongo/options")func main() {// Set up a connection to the MongoDB Atlas clusterclient, err := mongo.Connect(context.TODO(), options.Client().ApplyURI("mongodb+srv://:@/?retryWrites=true&w=majority"))if err != nil {log.Fatal(err)}defer client.Disconnect(context.TODO())// Get a handle to the "tasks" collection in the databasecollection := client.Database("task-manager").Collection("tasks")// Insert a new task into the collection_, err = collection.InsertOne(context.TODO(), bson.D{{"name", "Task 1"}, {"description", "This is the first task"}})if err != nil {log.Fatal(err)}// Find all tasks in the collectioncursor, err := collection.Find(context.TODO(), bson.D{})if err != nil {log.Fatal(err)}// Iterate over the results and print each taskfor cursor.Next(context.TODO()) {var task bson.Mif err := cursor.Decode(&task); err != nil {log.Fatal(err)}fmt.Println(task)}if err := cursor.Err(); err != nil {log.Fatal(err)}}
MongoDB Atlas has gained popularity due to its ease of use, scalability, and integration with other cloud services. It offers a variety of features that make it suitable for a wide range of applications, including real-time analytics, mobile app development, and data warehousing.
In this article, we will delve deeper into the world of MongoDB Atlas and explore its capabilities and benefits. We will also provide practical examples and code snippets to help you get started with MongoDB Atlas in your Go projects.
Working with MongoDB Atlas in Golang Projects
In this article, we will explore two key aspects of Working with MongoDB Atlas in Golang Projects: Cloud Database Management:
- Scalability and Flexibility: MongoDB Atlas is a highly scalable and flexible cloud database solution that can easily handle growing data volumes and varying workloads. It offers a variety of features such as auto-scaling, sharding, and replication, which allow you to scale your database as per your needs.
- Cost-Effectiveness: MongoDB Atlas is a cost-effective cloud database solution that offers a pay-as-you-go pricing model. This means that you only pay for the resources that you use, which can significantly reduce your database costs.
These key aspects make MongoDB Atlas an ideal choice for a wide range of applications, from small-scale projects to large-scale enterprise applications. For example, MongoDB Atlas is being used by companies such as Uber, Airbnb, and PayPal to manage their massive databases.
Scalability and Flexibility
In the context of Working with MongoDB Atlas in Golang Projects: Cloud Database Management, scalability and flexibility are crucial for handling the dynamic nature of modern applications. As your application grows and the data volume increases, MongoDB Atlas can automatically scale up or down to meet the changing demands, ensuring optimal performance and preventing bottlenecks.
- Auto-scaling: MongoDB Atlas can automatically adjust the number of database instances based on the workload. This ensures that your database has the resources it needs to handle peak loads without sacrificing performance during low-traffic periods.
- Sharding: MongoDB Atlas allows you to distribute your data across multiple servers, known as shards. This helps improve performance and scalability by reducing the load on any single server.
- Replication: MongoDB Atlas provides built-in replication, which means that your data is automatically replicated across multiple servers. This ensures high availability and data durability, reducing the risk of data loss in case of a hardware failure.
By leveraging these scalability and flexibility features, you can build robust and scalable Go applications that can handle varying workloads and data volumes, ensuring a seamless user experience and optimal application performance.
Cost-Effectiveness
In the context of Working with MongoDB Atlas in Golang Projects: Cloud Database Management, cost-effectiveness is a crucial factor for businesses looking to optimize their IT infrastructure and reduce operational costs.
By leveraging MongoDB Atlas’s pay-as-you-go pricing model, developers and businesses can avoid the upfront costs associated with traditional on-premises database deployments. This model allows you to scale your database resources as needed, without having to overprovision or pay for resources that you don’t use.
For example, a small startup with a limited budget can start with a basic MongoDB Atlas cluster and gradually scale up as their application and user base grows. This flexibility and cost-effectiveness make MongoDB Atlas an attractive option for startups and small businesses with limited resources.
Furthermore, MongoDB Atlas offers a variety of features that can help you further optimize your database costs. For instance, you can use auto-scaling to automatically adjust the size of your cluster based on demand, ensuring that you’re only paying for the resources that you need.
By understanding the cost-effectiveness of MongoDB Atlas and utilizing its features effectively, you can significantly reduce your database costs and improve the overall ROI of your Go applications.
FAQs on Working with MongoDB Atlas in Golang Projects
In this FAQ section, we will address some common questions and misconceptions related to Working with MongoDB Atlas in Golang Projects: Cloud Database Management.
Question 1: What are the key benefits of using MongoDB Atlas for Go projects?
MongoDB Atlas offers several key benefits for Go projects, including scalability, flexibility, cost-effectiveness, and ease of integration. It provides a fully managed database service, eliminating the need for database administration tasks, and supports a variety of features such as auto-scaling, sharding, and replication, making it suitable for handling varying workloads and data volumes.
Question 2: How do I connect to a MongoDB Atlas cluster from a Go application?
Connecting to a MongoDB Atlas cluster from a Go application is straightforward. You can use the official MongoDB Go driver to establish a connection and perform database operations. The driver provides a comprehensive set of methods for CRUD (create, read, update, delete) operations, as well as support for advanced features like transactions and change streams.
Question 3: Can I use MongoDB Atlas with other cloud services?
Yes, MongoDB Atlas integrates seamlessly with other cloud services, such as AWS, Azure, and Google Cloud. This integration allows you to leverage the capabilities of these cloud platforms, such as object storage, compute, and analytics, in conjunction with your MongoDB database.
Question 4: What are the best practices for using MongoDB Atlas in production?
To ensure optimal performance and reliability of your MongoDB Atlas deployment in production, it is recommended to follow best practices such as implementing proper authentication and authorization mechanisms, monitoring your database metrics, and creating regular backups to protect against data loss.
Question 5: Where can I find more information and support for MongoDB Atlas?
MongoDB provides extensive documentation, tutorials, and community support for MongoDB Atlas. You can refer to the official MongoDB Atlas documentation, participate in community forums, or contact MongoDB support for any assistance you may need.
Summary: MongoDB Atlas offers a powerful and scalable cloud database solution for Go projects. It provides a range of features and benefits that can significantly enhance the performance, reliability, and cost-effectiveness of your applications.
Transition to the next section: In the next section, we will explore the practical aspects of working with MongoDB Atlas in Golang projects, including code examples and best practices.
Tips for Working with MongoDB Atlas in Golang Projects
In this tips section, we will provide practical advice and best practices for effectively working with MongoDB Atlas in Golang projects.
Tip 1: Leverage MongoDB Atlas’s managed services
MongoDB Atlas is a fully managed database service, which means that you don’t have to worry about the underlying infrastructure and database administration tasks. This allows you to focus on developing your application and leave the database management to MongoDB Atlas.
Tip 2: Use the official MongoDB Go driver
The official MongoDB Go driver provides a comprehensive set of methods for CRUD (create, read, update, delete) operations, as well as support for advanced features like transactions and change streams. Using the official driver ensures compatibility and performance.
Tip 3: Implement proper authentication and authorization
Securing your MongoDB Atlas database is crucial. Implement strong authentication mechanisms, such as using MongoDB’s built-in role-based access control (RBAC), to control access to your database and prevent unauthorized changes.
Tip 4: Monitor your database metrics
Monitoring your MongoDB Atlas database metrics, such as performance, storage usage, and operation counts, is essential for ensuring optimal performance and identifying potential issues. Use MongoDB Atlas’s monitoring tools or third-party monitoring solutions to keep track of your database’s health.
Tip 5: Create regular backups
Protecting your data from loss is critical. MongoDB Atlas provides built-in backup and restore functionality. Regularly create backups of your database to a separate location to ensure data recovery in case of any incidents.
Summary: By following these tips, you can effectively leverage MongoDB Atlas in your Golang projects, ensuring data security, performance, and reliability.
Conclusion
In this article, we have explored the key aspects of Working with MongoDB Atlas in Golang Projects: Cloud Database Management. We have discussed the benefits of using MongoDB Atlas, including its scalability, flexibility, cost-effectiveness, and ease of integration with Go applications.
We have also provided practical tips and best practices for working with MongoDB Atlas in Golang projects. By following these tips, you can effectively leverage MongoDB Atlas to build scalable, performant, and reliable database-driven Go applications.
MongoDB Atlas is a powerful and versatile cloud database solution that can significantly enhance the capabilities of your Go projects. With its fully managed services, comprehensive Go driver support, and robust security features, MongoDB Atlas empowers you to focus on building innovative and impactful applications without the burden of database administration.
Youtube Video:
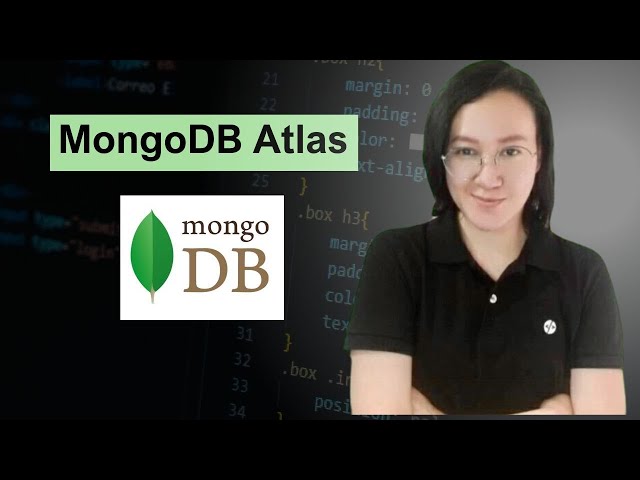