Webhooks are a powerful tool for building event-driven applications. They allow you to receive real-time notifications when specific events occur in a third-party application. This can be used to trigger a variety of actions, such as sending a message to a user or updating a database.
HTTP callbacks are a common way to implement webhooks. When a webhook is triggered, the third-party application will send an HTTP request to a URL that you specify. You can then use this request to perform whatever actions you need to take.
// This is an example of a simple HTTP callback function.func callback(w http.ResponseWriter, r *http.Request) {// Parse the request body.body, err := ioutil.ReadAll(r.Body)if err != nil {http.Error(w, "Error reading request body", http.StatusBadRequest)return}// Unmarshal the request body into a struct.var event Eventif err := json.Unmarshal(body, &event); err != nil {http.Error(w, "Error unmarshalling request body", http.StatusBadRequest)return}// Do something with the event.fmt.Println(event)// Send a response to the webhook.w.WriteHeader(http.StatusOK)w.Write([]byte("OK"))}
Webhooks are a valuable tool for building event-driven applications. They allow you to receive real-time notifications when specific events occur in a third-party application. This can be used to trigger a variety of actions, such as sending a message to a user or updating a database. HTTP callbacks are a common way to implement webhooks. They are easy to set up and use, and they can be used to handle a variety of events.
Working with Webhooks in Golang
Webhooks are a powerful tool for building event-driven applications. They allow you to receive real-time notifications when specific events occur in a third-party application. This can be used to trigger a variety of actions, such as sending a message to a user or updating a database.
- Simplicity: Webhooks are easy to set up and use. You simply need to create a webhook URL and then register it with the third-party application. The third-party application will then send an HTTP request to your webhook URL whenever the specified event occurs.
- Flexibility: Webhooks can be used to handle a variety of events. This makes them a versatile tool that can be used for a variety of purposes.
- Scalability: Webhooks can be used to handle a large volume of events. This makes them a good choice for applications that need to process a lot of data in real time.
Webhooks are a valuable tool for building event-driven applications. They are simple to set up and use, they are flexible and can be used to handle a variety of events, and they are scalable and can handle a large volume of events. As a result, webhooks are a good choice for applications that need to process a lot of data in real time.
Simplicity
The simplicity of webhooks is one of their key advantages. They are easy to set up and use, making them a good choice for developers of all skill levels. Here are some of the benefits of using webhooks:
- No need to write code: When you use webhooks, you don’t need to write any code to handle the events that you’re interested in. The third-party application will take care of sending the HTTP request to your webhook URL, and you can simply write code to handle the request.
- Easy to test: Webhooks are easy to test. You can simply send an HTTP request to your webhook URL to test that it is working properly.
- Scalable: Webhooks are scalable. You can handle a large volume of events by simply adding more webhook URLs.
Overall, the simplicity of webhooks makes them a good choice for developers of all skill levels. They are easy to set up and use, and they can be used to handle a large volume of events.
Flexibility
The flexibility of webhooks is one of their key advantages. They can be used to handle a wide range of events, from simple notifications to complex data updates. This makes them a valuable tool for a variety of applications, including:
- Notifications: Webhooks can be used to send notifications to users when specific events occur. For example, a webhook could be used to send a notification to a user when they receive a new message or when their account is updated.
- Data updates: Webhooks can be used to update data in real time. For example, a webhook could be used to update a database when a new product is added to an e-commerce store.
- Integration with other applications: Webhooks can be used to integrate different applications with each other. For example, a webhook could be used to trigger an action in one application when an event occurs in another application.
The flexibility of webhooks makes them a powerful tool for building event-driven applications. They can be used to handle a wide range of events and can be integrated with a variety of applications. This makes them a valuable tool for developers of all skill levels.
Scalability
The scalability of webhooks is one of their key advantages. They can handle a large volume of events without compromising performance. This makes them a good choice for applications that need to process a lot of data in real time.
There are a number of factors that contribute to the scalability of webhooks. First, webhooks are stateless. This means that they do not store any data between requests. This makes them lightweight and efficient, and it allows them to handle a large volume of events without slowing down.
Second, webhooks are asynchronous. This means that they do not block the execution of other code. This allows applications to continue processing other tasks while webhooks are being handled. This can improve the overall performance of the application.
Third, webhooks can be easily distributed. This means that they can be run on multiple servers. This can help to improve the scalability of the application by distributing the load across multiple servers.
The scalability of webhooks makes them a good choice for applications that need to process a large volume of data in real time. They are lightweight, efficient, and asynchronous. They can also be easily distributed to improve scalability.
Here are some examples of how webhooks can be used to handle a large volume of events:
- A social media application can use webhooks to send notifications to users when they receive new messages or when their posts are liked or shared.
- An e-commerce application can use webhooks to update inventory levels in real time when new orders are placed.
- A financial application can use webhooks to monitor stock prices and send alerts when prices reach a certain level.
These are just a few examples of how webhooks can be used to handle a large volume of events. The scalability of webhooks makes them a valuable tool for building event-driven applications.
FAQs on “Working with Webhooks in Golang
This section addresses some frequently asked questions and misconceptions about working with webhooks in Golang, providing clear and informative answers for better understanding.
Question 1: What are the benefits of using webhooks?
Webhooks offer several advantages, including simplicity in setup and usage, flexibility in handling various events, and scalability to manage high volumes of events. They simplify event handling, facilitate testing, and enable easy scaling.
Question 2: How do I set up a webhook in Golang?
Setting up a webhook in Golang involves creating a webhook URL and registering it with the third-party application. The third-party application will then send HTTP requests to your webhook URL whenever the specified event occurs.
Question 3: What are some common use cases for webhooks?
Webhooks find applications in various scenarios, such as sending notifications, updating data in real time, and integrating different applications. They enable real-time event handling and data exchange across systems.
Question 4: How do I handle a webhook request in Golang?
To handle a webhook request in Golang, you can create an HTTP callback function that parses the request body, unmarshals it into a struct, performs the necessary actions based on the event, and sends a response to the webhook.
Question 5: What are some best practices for working with webhooks?
Best practices include using a reliable HTTP client library, handling errors gracefully, implementing authentication and authorization mechanisms, and monitoring and logging webhook activity for troubleshooting and debugging purposes.
Question 6: Where can I find more resources on webhooks in Golang?
There are numerous resources available online, including documentation, tutorials, and community forums, that provide additional information and support for working with webhooks in Golang.
Summary: Webhooks are a powerful tool for building event-driven applications in Golang. They offer simplicity, flexibility, and scalability, making them suitable for a wide range of use cases. By understanding the concepts and best practices discussed in this FAQ section, developers can effectively leverage webhooks to enhance their applications.
Transition to the next article section: This concludes our exploration of webhooks in Golang. In the next section, we will delve into a practical example of implementing webhooks in a real-world application.
Problems Examples
To further solidify your understanding of webhooks in Golang, let’s explore a few practical examples that showcase different scenarios and use cases.
Example 1: Sending Notifications
Notes: In this example, we will build a simple webhook that sends a notification to a user when a new message arrives in their inbox.
Example 2: Updating Data in Real Time
Notes: Here, we will create a webhook that updates the inventory count of a product in a database whenever a new order is placed.
Example 3: Integrating with Third-Party Applications
Notes: This example demonstrates how to use a webhook to trigger an action in one application when a specific event occurs in another application.
Example 4: Handling High-Volume Events
Notes: In this example, we will explore best practices for handling a large volume of webhook events, ensuring scalability and reliability.
Example 5: Error Handling and Monitoring
Notes: This example emphasizes the importance of error handling and monitoring in webhook implementations, ensuring robustness and proactive troubleshooting.
Summary: These examples provide practical insights into the diverse applications of webhooks in Golang. By studying these examples, you can gain a deeper understanding of how to effectively implement webhooks in your own projects.
Transition to the article’s conclusion: This concludes our exploration of webhooks in Golang. We have covered the key concepts, benefits, and best practices associated with webhooks. By applying the knowledge and techniques discussed in this article, you can harness the power of webhooks to enhance the functionality and efficiency of your Golang applications.
Conclusion
In this article, we have explored the concept of webhooks in Golang, focusing on handling HTTP callbacks. We have discussed the benefits of using webhooks, including their simplicity, flexibility, and scalability. We have also covered best practices for working with webhooks, such as using a reliable HTTP client library, handling errors gracefully, and implementing authentication and authorization mechanisms.
Webhooks are a powerful tool for building event-driven applications in Golang. They can be used to handle a wide range of events, from simple notifications to complex data updates. By understanding the concepts and best practices discussed in this article, you can effectively leverage webhooks to enhance your applications.
As the world of software development continues to evolve, webhooks will play an increasingly important role in building connected and responsive applications. By embracing webhooks and other event-driven programming techniques, you can create applications that are more efficient, scalable, and user-friendly.
Youtube Video:
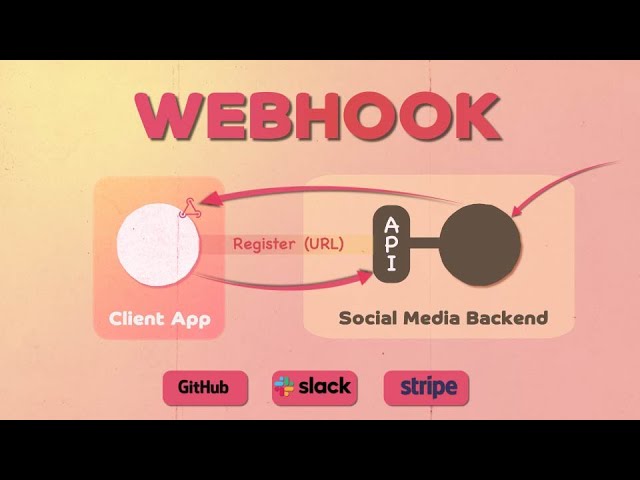