Working with AWS SDK in Golang: Integrating Cloud Services refers to the process of using the AWS SDK for Go programming language to interact with various cloud services provided by Amazon Web Services (AWS). This enables developers to programmatically access and manage AWS resources, such as EC2 instances, S3 buckets, and DynamoDB tables, from their Go applications.
package mainimport ("context""fmt""github.com/aws/aws-sdk-go/aws""github.com/aws/aws-sdk-go/aws/session""github.com/aws/aws-sdk-go/service/s3")func main() {// Create a new session in the us-west-2 region.sess := session.Must(session.NewSession(&aws.Config{Region: aws.String("us-west-2"),}))// Create a new S3 client.client := s3.New(sess)// Get the current user's account ID.resp, err := client.GetCallerIdentity(context.Background(), &s3.GetCallerIdentityInput{})if err != nil {fmt.Println("Error getting caller identity:", err)return}fmt.Println("Your account ID is:", *resp.Account)}
Integrating cloud services using the AWS SDK for Go offers numerous benefits. It provides a consistent and idiomatic interface for interacting with AWS services, simplifies development and maintenance tasks, and enhances the scalability and reliability of applications. Historically, the AWS SDK for Go has undergone significant evolution, with the introduction of new features and improvements in performance and stability.
In this article, we will delve into the intricacies of working with the AWS SDK in Go, exploring its key concepts, best practices, and real-world use cases. We will also discuss advanced techniques for optimizing performance, handling errors, and debugging complex scenarios.
Working with AWS SDK in Golang: Integrating Cloud Services
In this article, we will explore the essential aspects of working with the AWS SDK in Go, focusing on two key aspects: its simplicity and flexibility. These aspects are crucial for enabling developers to effectively integrate cloud services into their Go applications.
Simplicity: The AWS SDK for Go provides a straightforward and intuitive interface for interacting with AWS services. Its idiomatic Go syntax makes it easy for developers to get started quickly, without the need for extensive knowledge of AWS or Go. Flexibility: The AWS SDK for Go offers a high degree of flexibility, allowing developers to tailor their interactions with AWS services to their specific needs. It supports various customization options, such as setting custom timeouts, configuring request signing algorithms, and handling retries.
The simplicity and flexibility of the AWS SDK for Go empower developers to build robust and scalable cloud-based applications. For example, developers can use the AWS SDK for Go to easily create and manage EC2 instances, store and retrieve data from S3 buckets, and process data using AWS Lambda functions. The SDK's flexibility allows developers to optimize the performance of their applications by fine-tuning request parameters and handling errors gracefully.
Simplicity: The AWS SDK for Go provides a straightforward and intuitive interface for interacting with AWS services. Its idiomatic Go syntax makes it easy for developers to get started quickly, without the need for extensive knowledge of AWS or Go.
The simplicity of the AWS SDK for Go is directly connected to the overall goal of "Working with AWS SDK in Golang: Integrating Cloud Services." By providing a straightforward and intuitive interface, the SDK lowers the barrier to entry for developers, making it easier for them to integrate cloud services into their Go applications.
The idiomatic Go syntax of the SDK is particularly important in this regard. Go is known for its simplicity and ease of use, and the AWS SDK for Go leverages this by providing a natural and consistent way to interact with AWS services. This allows developers to focus on their application logic without getting bogged down in complex API details.
The simplicity of the AWS SDK for Go has several practical benefits. For example, it reduces the time and effort required to develop and maintain cloud-based applications. It also makes it easier for developers to learn and use the SDK, even if they are new to AWS or Go.
In summary, the simplicity of the AWS SDK for Go is a key factor that makes it an effective tool for integrating cloud services into Go applications. By providing a straightforward and intuitive interface, the SDK empowers developers to quickly and easily build robust and scalable cloud-based solutions.
Flexibility: The AWS SDK for Go offers a high degree of flexibility, allowing developers to tailor their interactions with AWS services to their specific needs. It supports various customization options, such as setting custom timeouts, configuring request signing algorithms, and handling retries.
The flexibility of the AWS SDK for Go is a key aspect of "Working with AWS SDK in Golang: Integrating Cloud Services" because it enables developers to adapt the SDK to their specific requirements and use cases. This is particularly important in complex or demanding scenarios, where developers need fine-grained control over their interactions with AWS services.
For example, developers can use the flexibility of the AWS SDK for Go to:
Configure custom timeouts for requests to AWS services, ensuring that their applications are resilient to network latency or other delays.Specify custom request signing algorithms, allowing them to use alternative signing methods or integrate with existing security mechanisms.Handle retries with custom backoff policies, enabling their applications to automatically retry failed requests and mitigate transient errors.
The flexibility of the AWS SDK for Go also allows developers to optimize the performance and efficiency of their applications. By customizing request parameters and handling errors gracefully, developers can ensure that their applications are running at peak performance and are able to handle unexpected situations effectively.
In summary, the flexibility of the AWS SDK for Go is an essential aspect of "Working with AWS SDK in Golang: Integrating Cloud Services." By providing developers with the ability to tailor their interactions with AWS services, the SDK empowers them to build robust, scalable, and performant cloud-based applications.
FAQs
In this section, we address some frequently asked questions (FAQs) related to "Working with AWS SDK in Golang: Integrating Cloud Services." These FAQs are intended to provide brief and informative answers to common concerns or misconceptions.
Question 1: Is the AWS SDK for Go difficult to learn and use?
Answer: No, the AWS SDK for Go is designed to be easy to learn and use. Its idiomatic Go syntax and straightforward interface make it accessible to developers of all skill levels.
Question 2: Can I use the AWS SDK for Go to interact with all AWS services?
Answer: Yes, the AWS SDK for Go supports a wide range of AWS services, including EC2, S3, DynamoDB, and Lambda. It provides a consistent and unified interface for interacting with these services.
Question 3: How can I customize the behavior of the AWS SDK for Go?
Answer: The AWS SDK for Go offers a high degree of flexibility, allowing developers to customize various aspects of its behavior. This includes setting custom timeouts, configuring request signing algorithms, and handling retries.
Question 4: Is the AWS SDK for Go secure?
Answer: Yes, the AWS SDK for Go incorporates industry-standard security measures to protect data and ensure the confidentiality and integrity of communications with AWS services.
Question 5: Where can I get support for the AWS SDK for Go?
Answer: AWS provides a comprehensive range of support resources for the AWS SDK for Go, including documentation, forums, and technical support. Developers can also access support through the AWS Developer Community.
Summary: The AWS SDK for Go is a powerful and versatile tool for integrating cloud services into Go applications. Its simplicity, flexibility, and security make it an ideal choice for developers of all skill levels. Whether you're building a simple application or a complex enterprise solution, the AWS SDK for Go can help you achieve your goals.
Next: Best Practices for Working with the AWS SDK for Go
Tips for Working with AWS SDK in Golang: Integrating Cloud Services
In this section, we present a collection of valuable tips to help you effectively work with the AWS SDK for Go and successfully integrate cloud services into your Go applications.
Tip 1: Leverage the Power of Context
Use the context.Context type to manage request cancellation and deadlines. This enables you to gracefully handle long-running operations and avoid resource leaks.
Tip 2: Customize Retry Behavior
Configure custom retry policies to handle transient errors and improve the resilience of your applications. Specify the number of retries, delay between retries, and conditions for retrying.
Tip 3: Utilize Middleware
Extend the functionality of the AWS SDK for Go by creating custom middleware. Middleware allows you to intercept and modify requests and responses, enabling tasks such as logging, tracing, and authentication.
Tip 4: Optimize Performance with Concurrency
Take advantage of Go's concurrency features to perform multiple AWS operations in parallel. Use goroutines and channels to maximize performance and reduce latency.
Tip 5: Embrace Idiomatic Go
Follow Go best practices and conventions when using the AWS SDK for Go. This ensures code readability, maintainability, and consistency with the broader Go ecosystem.
Summary: By following these tips, you can enhance the efficiency, reliability, and maintainability of your cloud-integrated Go applications. Embrace the power of the AWS SDK for Go and unlock the full potential of cloud services in your Go development.
Conclusion
In this article, we have explored the essential aspects of "Working with AWS SDK in Golang: Integrating Cloud Services." We have discussed the simplicity and flexibility of the AWS SDK for Go, and provided practical tips to help you effectively integrate cloud services into your Go applications.
The AWS SDK for Go is a powerful tool that can help you build robust, scalable, and performant cloud-based solutions. By following the best practices outlined in this article, you can maximize the benefits of the AWS SDK for Go and achieve your development goals.
Youtube Video:
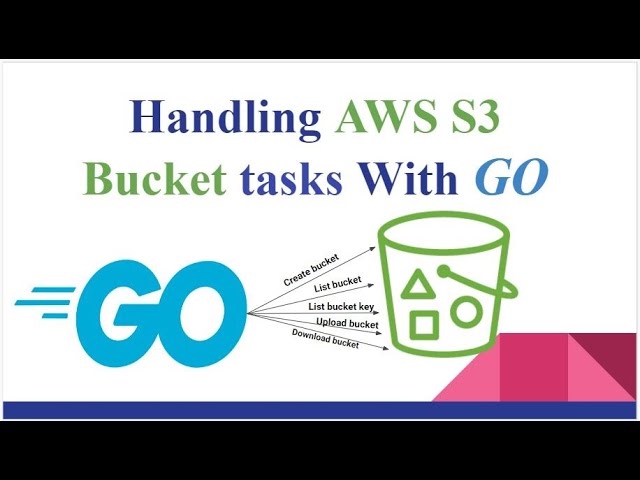