Redis is an in-memory data structure store, used as a database, cache, and message broker. It supports various data structures such as strings, hashes, lists, sets, sorted sets, and bitmaps. Redis can be used to implement caching, queuing, leaderboards, and other use cases.
// Import the necessary libraries.import ("context""fmt""github.com/go-redis/redis/v8")// Connect to the Redis server.rdb := redis.NewClient(&redis.Options{Addr: "localhost:6379",Password: "", // no password setDB: 0, // use default DB})// Set a key-value pair in Redis.err := rdb.Set(context.Background(), "name", "Redis", 0).Err()if err != nil {panic(err)}// Get the value associated with the key from Redis.val, err := rdb.Get(context.Background(), "name").Result()if err != nil {panic(err)}// Print the value.fmt.Println(val)// Close the Redis connection.defer rdb.Close()
Redis is highly performant, scalable, and reliable. It is open source and has a large community of users and contributors. Redis has been used in production by many large companies, including Twitter, Facebook, and Google.
In this article, we will discuss the basics of using Redis with Go, including how to connect to a Redis server, set and get keys, and use Redis for caching and pub/sub messaging.
Using Redis with Golang
Redis is a powerful in-memory data structure store that can be used for a variety of purposes, including caching and pub/sub messaging. It is highly performant, scalable, and reliable, making it a popular choice for many large companies.
- Caching: Redis can be used to cache data that is frequently accessed, such as the results of database queries or API calls. This can improve the performance of your application by reducing the number of times that it needs to access the slower backend store.
- Pub/Sub messaging: Redis can be used to implement a pub/sub messaging system, which allows you to send messages from one part of your application to another. This can be useful for a variety of purposes, such as sending notifications, triggering events, or updating data in real time.
These are just two of the many ways that Redis can be used with Golang. By leveraging the power of Redis, you can improve the performance, scalability, and reliability of your applications.
Caching
Caching is a technique that can be used to improve the performance of your application by storing frequently accessed data in a faster-to-access location. This can be especially useful for data that is expensive to generate or retrieve, such as the results of database queries or API calls.
Redis is a popular choice for caching because it is highly performant, scalable, and reliable. It can be used to cache a variety of data types, including strings, hashes, lists, sets, sorted sets, and bitmaps.
Using Redis for caching can provide a number of benefits, including:
- Reduced latency: By caching frequently accessed data in Redis, you can reduce the latency of your application by avoiding the need to access the slower backend store.
- Increased throughput: By caching frequently accessed data in Redis, you can increase the throughput of your application by handling more requests per second.
- Improved scalability: By caching frequently accessed data in Redis, you can improve the scalability of your application by reducing the load on your backend store.
Here is an example of how you can use Redis for caching in Golang:
// Import the necessary libraries.import ("context""fmt""github.com/go-redis/redis/v8")// Connect to the Redis server.rdb := redis.NewClient(&redis.Options{Addr: "localhost:6379",Password: "", // no password setDB: 0, // use default DB})// Set a key-value pair in Redis.err := rdb.Set(context.Background(), "name", "Redis", 0).Err()if err != nil {panic(err)}// Get the value associated with the key from Redis.val, err := rdb.Get(context.Background(), "name").Result()if err != nil {panic(err)}// Print the value.fmt.Println(val)// Close the Redis connection.defer rdb.Close()
In this example, we are using Redis to cache the value of the “name” key. We first set the value of the key to “Redis” using the Set() method. Then, we get the value of the key using the Get() method. Finally, we print the value of the key to the console.
Caching is a powerful technique that can be used to improve the performance of your application. By using Redis for caching, you can reduce latency, increase throughput, and improve scalability.
Pub/Sub messaging
Pub/sub messaging is a communication pattern that allows you to send messages from one part of your application to another. This can be useful for a variety of purposes, such as sending notifications, triggering events, or updating data in real time.
-
Components
A pub/sub messaging system consists of three main components: publishers, subscribers, and a message broker. Publishers are responsible for sending messages to the message broker. Subscribers are responsible for receiving messages from the message broker. The message broker is responsible for routing messages from publishers to subscribers. -
Examples
Pub/sub messaging can be used in a variety of applications, such as:- Sending notifications to users
- Triggering events in response to certain actions
- Updating data in real time
-
Implications
Pub/sub messaging can have a number of benefits for your application, including:- Decoupling: Pub/sub messaging can help to decouple your application components, making them more independent and easier to maintain.
- Scalability: Pub/sub messaging can help to scale your application by allowing you to add or remove publishers and subscribers as needed.
- Reliability: Pub/sub messaging can help to ensure that your messages are delivered reliably, even if there are failures in your system.
Redis is a popular choice for implementing pub/sub messaging systems because it is high-performance, scalable, and reliable. Redis also provides a number of features that make it easy to implement pub/sub messaging, such as the ability to create channels and subscribe to channels.
FAQs on Using Redis with Golang
In this section, we will answer some of the most common questions about using Redis with Golang for caching and pub/sub messaging.
Question 1: What are the benefits of using Redis for caching?
Redis is a high-performance, scalable, and reliable in-memory data structure store. It is well-suited for caching because it can store data in memory, which is much faster than accessing data from a disk-based database. Redis also supports a variety of data structures, which makes it a flexible caching solution.
Question 2: What are the benefits of using Redis for pub/sub messaging?
Redis is a high-performance, scalable, and reliable message broker. It is well-suited for pub/sub messaging because it can handle a large volume of messages with low latency. Redis also supports a variety of message types, which makes it a flexible messaging solution.
Question 3: How do I get started with using Redis with Golang?
To get started with using Redis with Golang, you can follow these steps:
- Install the Redis client library for Golang.
- Create a Redis client.
- Connect to a Redis server.
- Start using Redis for caching or pub/sub messaging.
Question 4: What are some best practices for using Redis with Golang?
Here are some best practices for using Redis with Golang:
- Use the appropriate data structure for your data.
- Set expiration times for your cached data.
- Use a connection pool to manage your Redis connections.
- Monitor your Redis usage.
Question 5: Where can I learn more about using Redis with Golang?
There are a number of resources available to help you learn more about using Redis with Golang, including the official Redis documentation, the Go Redis client library documentation, and various tutorials and blog posts.
We hope this FAQ section has been helpful. If you have any other questions, please feel free to contact us.
Next, we will discuss how to use Redis for caching in Golang.
Tips for Using Redis with Golang
In this section, we will provide some tips to help you get the most out of using Redis with Golang for caching and pub/sub messaging.
Tip 1: Choose the appropriate data structure for your data
Redis supports a variety of data structures, including strings, hashes, lists, sets, sorted sets, and bitmaps. It is important to choose the appropriate data structure for your data in order to optimize performance and efficiency.
Tip 2: Set expiration times for your cached data
If your cached data is not going to change frequently, you can set an expiration time for it. This will help to improve the performance of your application by reducing the number of times that it needs to access the backend store.
Tip 3: Use a connection pool to manage your Redis connections
Creating and destroying Redis connections can be expensive. To improve the performance of your application, you can use a connection pool to manage your Redis connections.
Tip 4: Monitor your Redis usage
It is important to monitor your Redis usage to ensure that you are not exceeding your limits. Redis provides a number of tools to help you monitor your usage, such as the INFO command and the Redis Dashboard.
Tip 5: Learn more about Redis
There are a number of resources available to help you learn more about Redis, including the official Redis documentation, the Go Redis client library documentation, and various tutorials and blog posts.
By following these tips, you can improve the performance, scalability, and reliability of your Redis applications.
Conclusion
In this article, we have explored the basics of using Redis with Golang for caching and pub/sub messaging. We have discussed the benefits of using Redis for these purposes, and we have provided some tips to help you get the most out of using Redis with Golang.
Redis is a powerful tool that can be used to improve the performance, scalability, and reliability of your applications. By leveraging the power of Redis, you can build applications that are able to handle a high volume of traffic and data.
Youtube Video:
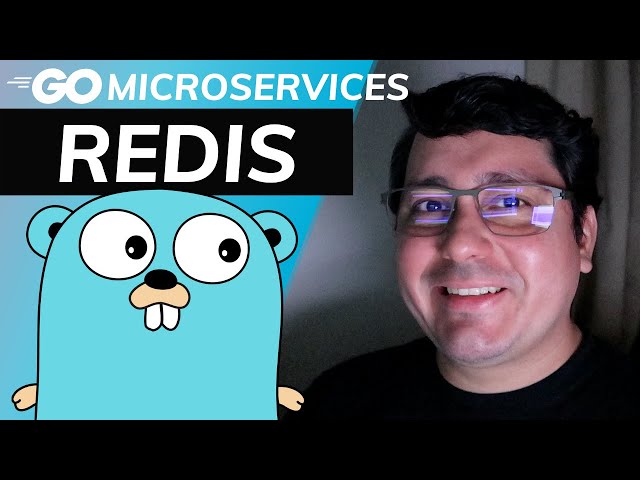