NATS Streaming is a powerful open-source streaming platform that enables the building of scalable, reliable, and fault-tolerant messaging systems. It is written in Go and provides a variety of features that make it ideal for building high-performance applications, including:
// Import the NATS Streaming client libraryimport nats "github.com/nats-io/nats-streaming-server/server"// Create a new NATS Streaming clientclient, err := nats.Connect("localhost:4222")if err != nil {log.Fatal(err)}// Create a new NATS Streaming connectionconn, err := client.Conn()if err != nil {log.Fatal(err)}// Publish a message to a NATS Streaming subjectif err := conn.Publish("my-subject", []byte("Hello world!")); err != nil {log.Fatal(err)}
NATS Streaming is a valuable tool for building scalable messaging systems. Its features make it ideal for a variety of applications and its popularity is growing rapidly. In this article, we will explore the basics of NATS Streaming and how to use it to build scalable messaging systems.
In this article, we will discuss the benefits of using NATS Streaming, provide a detailed overview of its features, and walk through a step-by-step tutorial on how to use NATS Streaming to build a scalable messaging system.
Using NATS Streaming with Golang
NATS Streaming is a powerful open-source streaming platform that enables the building of scalable, reliable, and fault-tolerant messaging systems. It is written in Go and provides a variety of features that make it ideal for building high-performance applications.
- Performance: NATS Streaming is designed to be performant, even at scale. It can handle millions of messages per second with low latency.
- Scalability: NATS Streaming is designed to be scalable, both horizontally and vertically. It can be deployed on a single server or across multiple servers to handle increasing demand.
- Reliability: NATS Streaming is designed to be reliable. It uses a variety of techniques, such as replication and persistence, to ensure that messages are delivered even in the event of failure.
These are just a few of the key aspects of NATS Streaming. In this article, we will explore these aspects in more detail and provide a step-by-step tutorial on how to use NATS Streaming to build a scalable messaging system.
Performance
Performance is a critical aspect of any messaging system. NATS Streaming is designed to be performant, even at scale. It can handle millions of messages per second with low latency. This makes it ideal for building applications that require high throughput and low latency.
There are a number of factors that contribute to NATS Streaming’s performance. First, NATS Streaming is written in Go, which is a high-performance language. Second, NATS Streaming uses a number of optimizations to improve performance, such as batching messages and using a binary protocol.
The performance of NATS Streaming has been proven in a number of real-world applications. For example, NATS Streaming is used by the New York Stock Exchange to power their market data platform. NATS Streaming is also used by a number of other large organizations, including Netflix, Uber, and Airbnb.
The performance of NATS Streaming is a key reason why it is a popular choice for building scalable messaging systems. NATS Streaming can handle the high throughput and low latency requirements of even the most demanding applications.
Scalability
Scalability is a critical aspect of any messaging system. NATS Streaming is designed to be scalable, both horizontally and vertically. This means that it can be deployed on a single server or across multiple servers to handle increasing demand.
Horizontal scalability refers to the ability to add more servers to a NATS Streaming cluster. This can be done to increase the throughput and capacity of the cluster. Vertical scalability refers to the ability to add more resources to a single NATS Streaming server. This can be done to improve the performance of the server.
The scalability of NATS Streaming is a key reason why it is a popular choice for building scalable messaging systems. NATS Streaming can be used to build systems that can handle the high throughput and low latency requirements of even the most demanding applications.
Here are some real-world examples of how NATS Streaming is used to build scalable messaging systems:
- The New York Stock Exchange uses NATS Streaming to power their market data platform.
- Netflix uses NATS Streaming to power their video streaming platform.
- Uber uses NATS Streaming to power their ride-sharing platform.
- Airbnb uses NATS Streaming to power their home-sharing platform.
These are just a few examples of how NATS Streaming is used to build scalable messaging systems. NATS Streaming is a powerful and versatile messaging platform that can be used to build a variety of scalable messaging systems.
Reliability
Reliability is a critical aspect of any messaging system. NATS Streaming is designed to be reliable, even in the event of failure. It uses a variety of techniques to achieve this, including replication and persistence.
- Replication: NATS Streaming replicates messages across multiple servers. This ensures that if one server fails, the messages will still be available on the other servers.
- Persistence: NATS Streaming persists messages to disk. This ensures that if a server fails, the messages will still be available after the server is restarted.
- Fault Tolerance: NATS Streaming is designed to be fault-tolerant. This means that it can continue to operate even if one or more servers fail.
The reliability of NATS Streaming is a key reason why it is a popular choice for building scalable messaging systems. NATS Streaming can be used to build systems that can handle the high throughput and low latency requirements of even the most demanding applications.
Using NATS Streaming with Golang
This FAQ section provides brief answers to common questions and misconceptions about using NATS Streaming with Golang.
Question 1: What are the benefits of using NATS Streaming?
Answer: NATS Streaming offers several benefits, including high performance, scalability, reliability, and fault tolerance.
Question 2: How do I get started with NATS Streaming and Golang?
Answer: You can find comprehensive documentation and tutorials on the NATS Streaming website.
Question 3: What are some real-world use cases for NATS Streaming?
Answer: NATS Streaming is used in various industries, including finance, media, and transportation.
Question 4: How can I contribute to the NATS Streaming community?
Answer: You can contribute by reporting issues, suggesting improvements, or participating in discussions.
Question 5: Where can I learn more about NATS Streaming?
Answer: The NATS Streaming website provides extensive resources, including documentation, tutorials, and community support.
These FAQs provide a concise overview of NATS Streaming and its capabilities. For more in-depth information, please refer to the official NATS Streaming documentation and resources.
Next: Building a Scalable Messaging System with NATS Streaming and Golang
Tips for Using NATS Streaming with Golang
In this section, we will provide some tips for using NATS Streaming with Golang. These tips will help you to build scalable and reliable messaging systems.
Tip 1: Use the NATS Streaming client library for Go
The NATS Streaming client library for Go provides a convenient and idiomatic way to interact with NATS Streaming. It is recommended to use this client library to develop your NATS Streaming applications.
Tip 2: Use a connection pool
When connecting to a NATS Streaming server, it is recommended to use a connection pool. This will help to improve the performance and reliability of your application.
Tip 3: Use subscriptions
Subscriptions allow you to receive messages from a NATS Streaming subject. It is recommended to use subscriptions to receive messages from multiple subjects in a scalable and efficient manner.
Tip 4: Use message persistence
Message persistence ensures that messages are not lost in the event of a server failure. It is recommended to use message persistence for important messages.
Tip 5: Monitor your NATS Streaming cluster
Monitoring your NATS Streaming cluster is essential for ensuring that it is running smoothly. There are a number of tools available for monitoring NATS Streaming, such as the NATS Streaming monitoring dashboard.
Conclusion
In this article, we have explored the basics of NATS Streaming and how to use it to build scalable messaging systems. We have discussed the performance, scalability, and reliability of NATS Streaming, and we have provided a number of tips for using NATS Streaming with Golang.
NATS Streaming is a powerful and versatile messaging platform that can be used to build a variety of scalable messaging systems. It is performant, scalable, reliable, and fault-tolerant. If you are looking for a messaging platform that can handle the high throughput and low latency requirements of your application, then NATS Streaming is a great option.
Youtube Video:
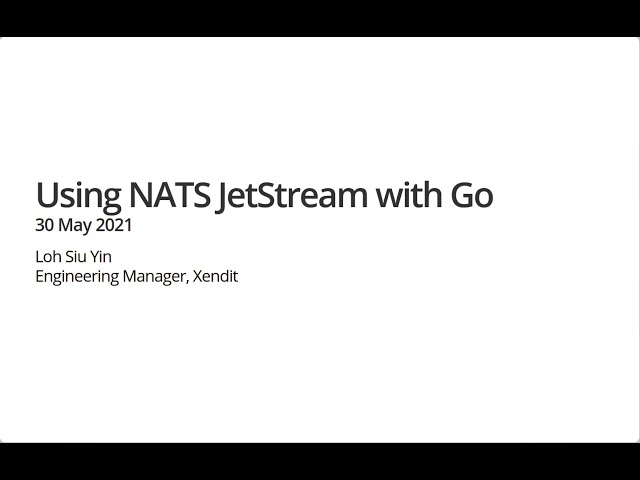