Using Kafka Streams with Golang: Processing Stream Data is a powerful open-source framework for building real-time data processing applications. It allows developers to easily create applications that can consume, transform, and produce data streams in real time.
// A simple Kafka Streams applicationpackage mainimport ( "context" "fmt" "time" "github.com/confluentinc/confluent-kafka-go/kafka")func main() { // Create a new Kafka consumer c, err := kafka.NewConsumer(&kafka.ConfigMap{ "bootstrap.servers": "localhost:9092", "group.id": "my-group", "auto.offset.reset": "earliest", }) if err != nil { panic(err) } // Subscribe to the "my-topic" topic c.SubscribeTopics([]string{"my-topic"}, nil) // Create a new channel to receive messages messages := make(chan *kafka.Message) // Start a goroutine to read messages from the Kafka consumer go func() { for { // Read the next message from the Kafka consumer msg, err := c.ReadMessage(-1) if err == nil { // Send the message to the messages channel messages <- msg } } }() // Process messages from the messages channel for msg := range messages { fmt.Printf("Received message: %s\n", string(msg.Value)) } // Wait for the Kafka consumer to close c.Close()}
Using Kafka Streams with Golang offers several benefits, including:
- High throughput and low latency
- Fault tolerance and scalability
- Ease of use and development
Kafka Streams was originally developed by LinkedIn in 2011 to power their real-time activity stream. It has since been open-sourced and adopted by many companies, including Uber, Airbnb, and Netflix.
In this article, we will explore the basics of Using Kafka Streams with Golang, including how to create a Kafka Streams application, how to consume and produce data streams, and how to use Kafka Streams to build real-time data processing applications.
Using Kafka Streams with Golang
Apache Kafka is a distributed streaming platform that enables you to build real-time data pipelines and applications. Kafka Streams is a library for building scalable, fault-tolerant stream processing applications on top of Kafka. It provides a high-level API for creating applications that can consume, transform, and produce data streams in real time.
- Scalability: Kafka Streams applications can be scaled out to handle large volumes of data. This is because Kafka Streams uses a distributed architecture that allows you to add or remove workers as needed.
- Fault tolerance: Kafka Streams applications are fault tolerant. This means that they can continue to process data even if one or more workers fail.
Kafka Streams is used in a variety of applications, including:
- Real-time data analytics
- Fraud detection
- Recommendation systems
- IoT data processing
Scalability
The scalability of Kafka Streams is one of its key advantages. This is because Kafka Streams uses a distributed architecture that allows you to add or remove workers as needed. This means that you can scale your Kafka Streams application to handle any . This is important for applications that need to process large volumes of data in real time.
-
Component: Distributed architecture
Kafka Streams uses a distributed architecture that allows you to add or remove workers as needed. This means that you can scale your Kafka Streams application to handle any . This is important for applications that need to process large volumes of data in real time.
- Example: A large e-commerce company uses Kafka Streams to process millions of orders per day. The company uses a distributed architecture to scale its Kafka Streams application to handle this large volume of data.
- Implication: The scalability of Kafka Streams makes it a good choice for applications that need to process large volumes of data in real time.
In addition to its scalability, Kafka Streams is also fault tolerant and easy to use. This makes it a good choice for a variety of applications.
Fault tolerance
Fault tolerance is a critical feature for any streaming application. It ensures that the application can continue to process data even if one or more of its workers fail. Kafka Streams provides fault tolerance by replicating data across multiple brokers. This means that if one broker fails, the other brokers can continue to serve data to the application.
The fault tolerance of Kafka Streams is essential for Using Kafka Streams with Golang: Processing Stream Data. This is because real-time data processing applications need to be able to handle failures without losing data. Kafka Streams provides this fault tolerance by ensuring that data is replicated across multiple brokers. This means that even if one broker fails, the other brokers can continue to serve data to the application.
For example, a large financial institution uses Kafka Streams to process millions of transactions per day. The institution uses Kafka Streams’ fault tolerance to ensure that the application can continue to process transactions even if one or more of its workers fail. This is critical for the institution because it needs to be able to process transactions in real time.
The fault tolerance of Kafka Streams is a key feature that makes it a good choice for building real-time data processing applications. Kafka Streams provides fault tolerance by replicating data across multiple brokers. This ensures that the application can continue to process data even if one or more of its workers fail.
Real-time data analytics
Real-time data analytics is the process of analyzing data as it is being generated. This is in contrast to traditional data analytics, which analyzes data that has already been collected and stored. Real-time data analytics is important because it allows businesses to make decisions based on the most up-to-date information available.
-
Component: Data sources
Real-time data analytics requires data sources that can provide data in real time. These data sources can include sensors, IoT devices, and social media feeds.
- Example: A manufacturing company uses real-time data analytics to monitor its production line. The company uses sensors to collect data on the speed of the production line, the temperature of the machines, and the quality of the products. This data is then analyzed in real time to identify potential problems and make adjustments to the production line.
- Implication: Real-time data analytics can help businesses to improve their efficiency and productivity.
-
Component: Stream processing
Real-time data analytics requires stream processing software to analyze data as it is being generated. This software can filter, aggregate, and transform data in real time.
- Example: A financial institution uses real-time data analytics to detect fraud. The institution uses stream processing software to analyze data on transactions as they are being made. This data is then analyzed in real time to identify potential fraud.
- Implication: Real-time data analytics can help businesses to reduce their risk of fraud.
Real-time data analytics is a powerful tool that can help businesses to make better decisions and improve their operations. Kafka Streams is a stream processing platform that can be used to build real-time data analytics applications. Kafka Streams is scalable, fault tolerant, and easy to use. This makes it a good choice for building real-time data analytics applications.
Fraud detection
Fraud detection is a critical application of Using Kafka Streams with Golang: Processing Stream Data. Fraudulent activities can result in significant financial losses for businesses, so it is important to be able to detect and prevent them in real time.
-
Title of Facet 1: Real-time data sources
To detect fraud in real time, it is important to have access to real-time data sources. These data sources can include transaction logs, sensor data, and social media feeds.
-
Title of Facet 2: Stream processing
Once you have access to real-time data sources, you need to use stream processing to analyze the data and identify potential fraud. Stream processing software can filter, aggregate, and transform data in real time.
-
Title of Facet 3: Machine learning
Machine learning can be used to develop models that can identify fraudulent activities. These models can be used to score transactions in real time and identify those that are most likely to be fraudulent.
-
Title of Facet 4: Actionable insights
Once you have identified potential fraudulent activities, you need to take action to prevent them. This could involve blocking the transaction, contacting the customer, or investigating the activity further.
Using Kafka Streams with Golang is a powerful tool for building fraud detection applications. Kafka Streams is scalable, fault tolerant, and easy to use. This makes it a good choice for building real-time fraud detection applications that can help businesses to protect themselves from financial losses.
Recommendation systems
Recommendation systems are a type of information filtering system that seeks to predict the rating or preference a user would give to an item. They are used in a variety of applications, such as recommending products to users on e-commerce websites, recommending movies to users on streaming services, and recommending articles to users on news websites.
Recommendation systems are typically built using machine learning algorithms. These algorithms are trained on a dataset of user-item interactions, such as ratings, purchases, or clicks. The algorithms learn to identify patterns in the data that can be used to predict user preferences. Once the algorithms are trained, they can be used to generate recommendations for new users or for new items.
Using Kafka Streams with Golang can be used to build real-time recommendation systems. Kafka Streams is a stream processing platform that can be used to process data in real time. This makes it a good choice for building recommendation systems, because it allows you to generate recommendations based on the most up-to-date data.
For example, a large e-commerce company could use Kafka Streams to build a real-time recommendation system. The company could use Kafka Streams to process data on user purchases and interactions in real time. This data could then be used to train a machine learning model that can predict user preferences. The model could then be used to generate recommendations for users in real time.
Recommendation systems are an important part of many online businesses. They can help businesses to increase sales and improve customer satisfaction. Using Kafka Streams with Golang is a powerful way to build real-time recommendation systems that can be used to improve the user experience and increase business revenue.
IoT data processing
The Internet of Things (IoT) is a network of physical devices that are connected to the internet and can collect and exchange data. IoT devices are used in a wide variety of applications, including smart homes, smart cities, and industrial automation. IoT data processing is the process of collecting, storing, and analyzing data from IoT devices.
-
Data sources
IoT devices generate a large amount of data, including sensor data, telemetry data, and event data. This data can be used to monitor the status of IoT devices, track the movement of objects, and identify trends. Using Kafka Streams with Golang can be used to collect and process data from IoT devices in real time.
-
Stream processing
IoT data is often processed in real time using stream processing software. Stream processing software can filter, aggregate, and transform data in real time. Using Kafka Streams with Golang can be used to process IoT data in real time and identify patterns and trends.
-
Machine learning
Machine learning can be used to develop models that can predict the behavior of IoT devices and identify anomalies. These models can be used to improve the efficiency of IoT devices and to identify potential problems.
-
Actionable insights
IoT data can be used to generate actionable insights that can help businesses to improve their operations. For example, IoT data can be used to identify inefficiencies in production processes, track the movement of assets, and predict the demand for products.
Using Kafka Streams with Golang is a powerful tool for building IoT data processing applications. Kafka Streams is scalable, fault tolerant, and easy to use. This makes it a good choice for building IoT data processing applications that can help businesses to improve their operations.
FAQs on “Using Kafka Streams with Golang
This section addresses common questions and misconceptions about using Kafka Streams with Golang for stream data processing.
Question 1: What are the benefits of using Kafka Streams with Golang for stream data processing?
Kafka Streams with Golang offers several benefits for stream data processing, including:
- Scalability: Kafka Streams can be easily scaled out to handle large volumes of data.
- Fault tolerance: Kafka Streams is fault tolerant, ensuring data processing continues even if some workers fail.
- Ease of use: Kafka Streams provides a user-friendly API for building stream processing applications.
Question 2: What are some common use cases for Kafka Streams with Golang?
Kafka Streams with Golang is used in various applications, including:
- Real-time data analytics
- Fraud detection
- Recommendation systems
- IoT data processing
These use cases demonstrate the versatility of Kafka Streams with Golang in handling different types of stream data.
Question 3: How does Kafka Streams with Golang handle data partitioning?
Kafka Streams with Golang uses partitioning to distribute data across multiple workers for parallel processing. Each partition is assigned to a specific worker, allowing for efficient load balancing and scalability.
Question 4: What are the key considerations for designing a Kafka Streams with Golang application?
Designing a Kafka Streams with Golang application involves considering factors such as data partitioning, topology design, and fault tolerance mechanisms. Proper planning and design ensure optimal performance and reliability.
Question 5: What resources are available for learning and support on Kafka Streams with Golang?
There are various resources available for learning and support, including the official Kafka documentation, tutorials, community forums, and training courses. These resources provide comprehensive guidance on Kafka Streams with Golang, enabling developers to build robust stream processing applications.
In summary, Kafka Streams with Golang is a powerful tool for building scalable, fault-tolerant, and efficient stream data processing applications. Its benefits and wide range of use cases make it a valuable choice for handling real-time data challenges.
Transition to the next article section: Exploring Advanced Features of Kafka Streams with Golang
Tips for Using Kafka Streams with Golang
To get the most out of Kafka Streams with Golang, consider these practical tips:
Tip 1: Leverage partitioning for scalability
Partitioning divides data into smaller chunks, enabling parallel processing across multiple workers. This optimizes throughput and scalability, especially for high-volume data streams.
Tip 2: Design a robust topology
The topology defines the flow of data through your Kafka Streams application. Plan the topology carefully, considering factors like data dependencies and processing requirements. A well-designed topology ensures efficient and reliable data processing.
Tip 3: Handle fault tolerance effectively
Kafka Streams provides fault tolerance mechanisms to ensure data integrity and application resilience. Implement these mechanisms, such as replication and state management, to handle worker failures and prevent data loss.
Tip 4: Utilize the Kafka Streams DSL
The Kafka Streams DSL (Domain-Specific Language) offers a concise and expressive way to define data transformations and processing logic. Leverage the DSL to simplify development and improve code readability.
Tip 5: Monitor and tune your application
Regularly monitor your Kafka Streams application to identify performance bottlenecks and areas for optimization. Use metrics and profiling tools to fine-tune configuration parameters and improve overall efficiency.
Conclusion
In this article, we explored the fundamentals and applications of using Kafka Streams with Golang for stream data processing. We discussed the benefits, key concepts, and considerations for building scalable, fault-tolerant, and efficient stream processing applications.
Kafka Streams with Golang empowers developers to harness the power of real-time data processing for various use cases, including real-time analytics, fraud detection, recommendation systems, and IoT data processing. By leveraging its features and implementing best practices, you can unlock the full potential of stream data processing and gain valuable insights to drive informed decision-making.
Youtube Video:
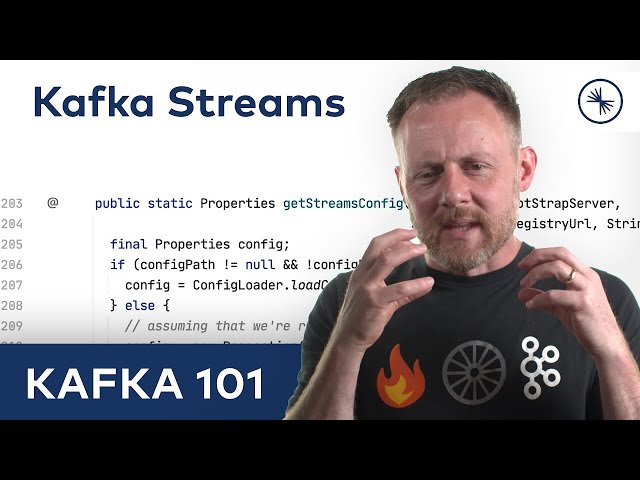