Gorilla Toolkit is a powerful web toolkit for building APIs in Golang. It provides a comprehensive set of features for creating robust, scalable, and efficient APIs.
package mainimport ("fmt""log""net/http""github.com/gorilla/mux")func main() {r := mux.NewRouter()r.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) {fmt.Fprintf(w, "Hello, World!")})log.Fatal(http.ListenAndServe(":8080", r))}
Gorilla Toolkit is particularly well-suited for building RESTful APIs. It provides a range of features that make it easy to create APIs that are compliant with the REST architectural style. These features include support for different HTTP methods, content negotiation, and resource representation.
Gorilla Toolkit has been widely adopted by the Golang community and is used in a number of popular open-source projects. It is a well-maintained and actively developed project, making it a reliable choice for building production-grade APIs.
Using Gorilla Toolkit in Golang
Gorilla Toolkit is a powerful web toolkit for building APIs in Golang. It provides a comprehensive set of features for creating robust, scalable, and efficient APIs. Three key aspects of Gorilla Toolkit are its ease of use, its extensibility, and its performance.
- Ease of use: Gorilla Toolkit is designed to be easy to use, even for developers who are new to Golang. It provides a simple and intuitive API that makes it easy to create APIs quickly and easily.
- Extensibility: Gorilla Toolkit is extensible, allowing developers to add their own functionality to the toolkit. This makes it possible to create custom APIs that meet the specific needs of a project.
- Performance: Gorilla Toolkit is designed to be performant, even under heavy load. It uses a number of techniques to improve performance, such as caching and concurrency.
These three aspects make Gorilla Toolkit an ideal choice for building APIs in Golang. It is easy to use, extensible, and performant.
Ease of use
The ease of use of Gorilla Toolkit is one of its key strengths. It is designed to be easy to learn and use, even for developers who are new to Golang. The toolkit provides a simple and intuitive API that makes it easy to create APIs quickly and easily. This ease of use is a major benefit for developers, as it allows them to focus on the business logic of their APIs, rather than on the underlying implementation details.
For example, Gorilla Toolkit provides a number of built-in features that make it easy to create RESTful APIs. These features include support for different HTTP methods, content negotiation, and resource representation. This means that developers do not need to write boilerplate code to implement these features, which can save them a significant amount of time and effort.
The ease of use of Gorilla Toolkit is also evident in the documentation and community support that is available. The toolkit is well-documented, with a number of tutorials and examples that can help developers get started. There is also a large and active community of Gorilla Toolkit users who are willing to help others. This makes it easy for developers to find the help and support that they need.
Overall, the ease of use of Gorilla Toolkit is a major benefit for developers. It makes it easy to create APIs quickly and easily, even for developers who are new to Golang.
Extensibility
The extensibility of Gorilla Toolkit is one of its key strengths. It allows developers to add their own functionality to the toolkit, making it possible to create custom APIs that meet the specific needs of a project.
-
Custom middleware
Middleware is a powerful feature of Gorilla Toolkit that allows developers to intercept and process requests and responses. Developers can use middleware to add custom functionality to their APIs, such as authentication, authorization, logging, and rate limiting. -
Custom routers
Gorilla Toolkit provides a powerful router that can be used to define the routes for an API. Developers can create custom routers to add custom functionality to the routing process, such as support for different HTTP methods, content negotiation, and resource representation. -
Custom handlers
Handlers are responsible for processing requests and generating responses. Developers can create custom handlers to add custom functionality to their APIs, such as support for different data formats, custom error handling, and custom response codes. -
Custom filters
Filters are used to modify the request and response objects before and after they are processed by the handlers. Developers can create custom filters to add custom functionality to their APIs, such as data validation, input sanitization, and output compression.
The extensibility of Gorilla Toolkit makes it a powerful tool for building custom APIs. Developers can use the toolkit to create APIs that meet the specific needs of their projects, without having to write boilerplate code or implement complex functionality from scratch.
Performance
The performance of an API is critical to its success. Users expect APIs to be fast and responsive, even under heavy load. Gorilla Toolkit is designed to be performant, even under heavy load. It uses a number of techniques to improve performance, such as caching and concurrency.
-
Caching
Caching is a technique that can be used to improve the performance of an API by storing the results of frequently executed queries in memory. This means that the API can avoid having to re-execute the query every time it is requested, which can improve performance significantly. -
Concurrency
Concurrency is a technique that can be used to improve the performance of an API by allowing multiple requests to be processed simultaneously. This can be achieved by using multiple threads or processes to handle requests. Gorilla Toolkit supports concurrency, which can help to improve the performance of APIs that are under heavy load.
The performance of Gorilla Toolkit is one of its key strengths. It is designed to be performant, even under heavy load. This makes it a good choice for building APIs that are expected to handle a large volume of traffic.
FAQs
This section addresses some common questions and misconceptions about using Gorilla Toolkit in Golang for building APIs.
Question 1: Is Gorilla Toolkit suitable for building production-grade APIs?
Answer: Yes, Gorilla Toolkit is a reliable and well-maintained toolkit that is used in production by many popular open-source projects.
Question 2: Is Gorilla Toolkit difficult to learn and use?
Answer: No, Gorilla Toolkit is designed to be easy to learn and use, even for developers who are new to Golang.
Question 3: Can Gorilla Toolkit be used to build custom APIs?
Answer: Yes, Gorilla Toolkit is extensible, allowing developers to add their own functionality to the toolkit. This makes it possible to create custom APIs that meet the specific needs of a project.
Question 4: Is Gorilla Toolkit performant?
Answer: Yes, Gorilla Toolkit is designed to be performant, even under heavy load. It uses a number of techniques to improve performance, such as caching and concurrency.
Question 5: Where can I find more information about Gorilla Toolkit?
Answer: The Gorilla Toolkit website (https://gorilla-web.readthedocs.io/en/stable/) provides comprehensive documentation, tutorials, and examples.
Summary: Gorilla Toolkit is a powerful and versatile web toolkit for building APIs in Golang. It is easy to learn and use, extensible, performant, and well-suited for building production-grade APIs.
Transition to the next article section: In the next section, we will discuss how to use Gorilla Toolkit to build a simple RESTful API.
Tips for Using Gorilla Toolkit in Golang
Here are a few tips for using Gorilla Toolkit to build APIs in Golang:
Tip 1: Use middleware to add custom functionality to your APIs.
Middleware is a powerful feature of Gorilla Toolkit that allows developers to intercept and process requests and responses. Developers can use middleware to add custom functionality to their APIs, such as authentication, authorization, logging, and rate limiting.
Tip 2: Use custom routers to add custom functionality to the routing process.
Gorilla Toolkit provides a powerful router that can be used to define the routes for an API. Developers can create custom routers to add custom functionality to the routing process, such as support for different HTTP methods, content negotiation, and resource representation.
Tip 3: Use custom handlers to add custom functionality to your APIs.
Handlers are responsible for processing requests and generating responses. Developers can create custom handlers to add custom functionality to their APIs, such as support for different data formats, custom error handling, and custom response codes.
Tip 4: Use custom filters to modify the request and response objects.
Filters are used to modify the request and response objects before and after they are processed by the handlers. Developers can create custom filters to add custom functionality to their APIs, such as data validation, input sanitization, and output compression.
Tip 5: Use the documentation and community support to get help.
Gorilla Toolkit is well-documented, with a number of tutorials and examples that can help developers get started. There is also a large and active community of Gorilla Toolkit users who are willing to help others. This makes it easy for developers to find the help and support that they need.
Summary: Gorilla Toolkit is a powerful and versatile web toolkit for building APIs in Golang. By following these tips, developers can use Gorilla Toolkit to build custom APIs that meet the specific needs of their projects.
Conclusion
In this article, we have explored the use of Gorilla Toolkit in Golang for building APIs. We have discussed the ease of use, extensibility, and performance of Gorilla Toolkit, and we have provided some tips for using Gorilla Toolkit to build custom APIs.
Gorilla Toolkit is a powerful and versatile tool for building APIs in Golang. It is easy to learn and use, extensible, performant, and well-suited for building production-grade APIs. Developers who are looking for a toolkit to help them build custom APIs should consider using Gorilla Toolkit.
Youtube Video:
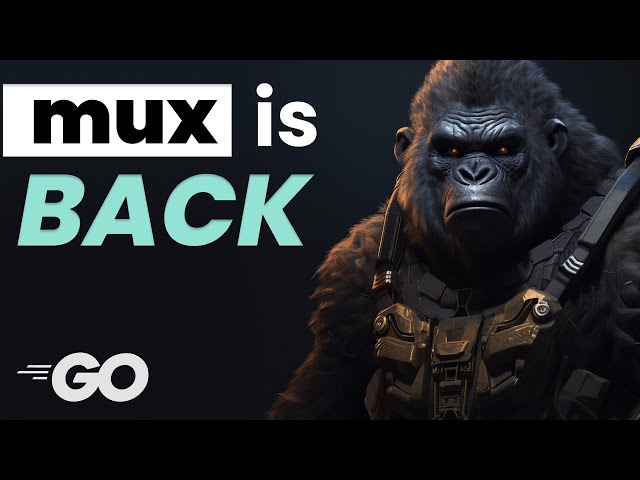