Event-driven architecture (EDA) is a software design pattern that emphasizes the use of events to trigger actions and facilitate communication between different parts of an application. In Golang, EDA can be implemented using channels, message queues, or event buses to pass messages between components.
One of the benefits of using EDA in Golang applications is that it can improve scalability and performance by decoupling the different components of the application. This allows each component to operate independently and at its own pace, without being affected by the performance of other components. Additionally, EDA can make it easier to develop and maintain complex applications by providing a clear and structured way to handle events.
// Create a channel to pass messages between components.channel := make(chan string)// Start a goroutine to listen for messages on the channel.go func() {for {message := <-channelfmt.Println(message)}}()// Send a message to the channel.channel <- "Hello, world!"
EDA is a powerful tool that can be used to improve the scalability, performance, and maintainability of Golang applications. By understanding the basics of EDA and how to implement it in your own applications, you can take advantage of its benefits and build more efficient and effective software.
Exploring Event-Driven Architecture in Golang Applications
Event-driven architecture (EDA) is a powerful design pattern that can be used to improve the scalability, performance, and maintainability of Golang applications. By understanding the key aspects of EDA and how to implement it in your own applications, you can take advantage of its benefits and build more efficient and effective software.
- Decoupling: EDA decouples the different components of an application, allowing them to operate independently and at their own pace.
- Scalability: EDA can improve the scalability of an application by making it easier to add new components or scale existing components independently.
- Performance: EDA can improve the performance of an application by reducing the amount of time spent on inter-component communication.
- Maintainability: EDA can make an application easier to maintain by providing a clear and structured way to handle events.
- Flexibility: EDA makes it easier to change the behavior of an application by adding or removing event handlers.
- Concurrency: EDA can be used to implement concurrent applications, which can improve the performance and scalability of an application.
These are just a few of the key aspects of EDA. By understanding these aspects and how to implement EDA in your own applications, you can take advantage of its benefits and build more efficient and effective software.
Decoupling
Decoupling is a key aspect of event-driven architecture (EDA). It refers to the practice of designing software systems in such a way that the different components are loosely coupled and can operate independently of each other.
- Benefits of decoupling: Decoupling can provide a number of benefits, including improved scalability, performance, and maintainability. By decoupling the different components of an application, it becomes easier to add new components or scale existing components independently. Additionally, decoupling can reduce the amount of time spent on inter-component communication, which can improve the performance of an application.
- How to achieve decoupling: There are a number of ways to achieve decoupling in EDA applications. One common approach is to use message queues or event buses to pass messages between components. This allows the different components to communicate with each other without having to be directly connected.
- Examples of decoupling in Golang: There are a number of Golang libraries and frameworks that can be used to implement decoupled EDA applications. Some popular options include the Go channels package, the NATS streaming platform, and the Apache Kafka distributed streaming platform.
Decoupling is an essential aspect of EDA and can provide a number of benefits for Golang applications. By understanding the benefits of decoupling and how to achieve it, you can design and develop more scalable, performant, and maintainable applications.
Scalability
In the context of event-driven architecture (EDA), scalability refers to the ability of an application to handle increasing loads or traffic without compromising performance. EDA can improve the scalability of an application by making it easier to add new components or scale existing components independently.
- Horizontal scalability: EDA enables horizontal scalability by allowing you to add new components to the application without affecting the existing components. This is because the components are loosely coupled and communicate with each other through events.
- Vertical scalability: EDA also enables vertical scalability by allowing you to scale existing components independently. This means that you can increase the capacity of a component without affecting the other components.
- Examples of scalability in Golang: There are a number of Golang libraries and frameworks that can be used to implement scalable EDA applications. Some popular options include the Go channels package, the NATS streaming platform, and the Apache Kafka distributed streaming platform.
Scalability is a key benefit of EDA and can be a major advantage for Golang applications. By understanding the benefits of scalability and how to achieve it, you can design and develop more scalable applications that can handle increasing loads or traffic without compromising performance.
Performance
In the context of event-driven architecture (EDA), performance refers to the ability of an application to respond to requests and events in a timely manner. EDA can improve the performance of an application by reducing the amount of time spent on inter-component communication.
- Asynchronous communication: EDA uses asynchronous communication to pass messages between components. This means that the sender of a message does not have to wait for the receiver to process the message before continuing. This can significantly improve the performance of an application, especially for applications that need to process a large number of messages.
- Concurrency: EDA can be used to implement concurrent applications. This means that multiple components can process messages at the same time. This can further improve the performance of an application, especially for applications that need to handle a high volume of traffic.
- Message queues: EDA often uses message queues to store messages that are waiting to be processed. Message queues can help to improve the performance of an application by buffering messages and ensuring that they are processed in a timely manner.
Improving the performance of an application is a key goal for any software engineer. By understanding the benefits of EDA and how to implement it in Golang applications, you can design and develop more performant applications that can handle increasing loads or traffic without compromising performance.
Maintainability
As software applications grow in size and complexity, it becomes increasingly important to design and develop them in a way that makes them easy to maintain. Event-driven architecture (EDA) can help to improve the maintainability of an application by providing a clear and structured way to handle events.
One of the key challenges of maintaining a complex application is managing the interactions between different components. In a traditional monolithic application, all of the components are tightly coupled and interdependent. This can make it difficult to make changes to one component without affecting the other components. EDA addresses this challenge by decoupling the different components of an application and allowing them to communicate with each other through events.
By decoupling the components of an application, EDA makes it easier to make changes to one component without affecting the other components. This can significantly reduce the time and effort required to maintain an application. Additionally, EDA can help to improve the reliability of an application by making it more resilient to failures in individual components.
Here is an example of how EDA can be used to improve the maintainability of an application: Consider an e-commerce application that uses a traditional monolithic architecture. The application consists of a number of different components, including a web server, a database, and a payment gateway. If the payment gateway component fails, the entire application will become unavailable. However, if the application were redesigned using EDA, the payment gateway component could be decoupled from the other components. This would mean that if the payment gateway component failed, the other components of the application would still be able to function.
EDA is a powerful tool that can be used to improve the maintainability, reliability, and scalability of Golang applications. By understanding the benefits of EDA and how to implement it in your own applications, you can design and develop more maintainable, reliable, and scalable applications.
Flexibility
Flexibility is a key aspect of event-driven architecture (EDA) that makes it easier to change the behavior of an application by adding or removing event handlers. This is because EDA decouples the different components of an application and allows them to communicate with each other through events. As a result, you can change the behavior of an application by simply adding or removing event handlers, without having to modify the code of the other components.
For example, consider an e-commerce application that uses EDA to handle orders. The application consists of a number of different components, including a web server, a database, and a shipping service. When an order is placed, the web server sends an event to the database to create a new order record. The database then sends an event to the shipping service to ship the order. If you want to change the behavior of the application, such as adding a new step to the order process, you can simply add a new event handler to the application. This will allow you to change the behavior of the application without having to modify the code of the other components.
Flexibility is an important aspect of EDA that makes it a good choice for developing complex applications that need to be able to change over time. By understanding the benefits of flexibility and how to implement it in Golang applications, you can design and develop more flexible applications that can be easily adapted to changing requirements.
In summary, EDA makes it easier to change the behavior of an application by adding or removing event handlers. This is because EDA decouples the different components of an application and allows them to communicate with each other through events. As a result, you can change the behavior of an application by simply adding or removing event handlers, without having to modify the code of the other components. Flexibility is an important aspect of EDA that makes it a good choice for developing complex applications that need to be able to change over time.
Concurrency
In the context of event-driven architecture (EDA), concurrency refers to the ability of an application to process multiple events or tasks simultaneously. This can be achieved by using multiple threads or processes to handle different events or tasks in parallel. Concurrency can improve the performance and scalability of an application by allowing it to handle more requests or events without compromising performance.
- Improved performance: By processing multiple events or tasks concurrently, an application can reduce the amount of time it takes to respond to requests or events. This can lead to a noticeable improvement in performance, especially for applications that need to handle a high volume of traffic.
- Increased scalability: Concurrency can also help to improve the scalability of an application by allowing it to handle more requests or events without compromising performance. This is because a concurrent application can scale horizontally by adding more threads or processes to handle the increased load.
- Examples of concurrency in Golang: There are a number of ways to implement concurrency in Golang applications. Some popular options include using the Go channels package, the Go concurrency patterns package, and the Go async/await feature.
Concurrency is a powerful tool that can be used to improve the performance and scalability of Golang applications. By understanding the benefits of concurrency and how to implement it in your own applications, you can design and develop more efficient and scalable applications.
FAQs on Exploring Event-Driven Architecture in Golang Applications
This section addresses frequently asked questions (FAQs) to provide a deeper understanding of event-driven architecture (EDA) in Golang applications.
Question 1: What are the key benefits of using EDA in Golang applications?
Answer: EDA offers several advantages, including improved scalability, performance, maintainability, flexibility, and concurrency. It decouples components, enabling independent operation and scaling. EDA enhances performance by reducing inter-component communication time. It promotes maintainability through structured event handling, making it easier to modify application behavior. Additionally, EDA provides flexibility by allowing for easy addition or removal of event handlers. Lastly, it supports concurrency, allowing for parallel processing of events, leading to improved performance and scalability.
Question 2: How does EDA improve the scalability of Golang applications?
Answer: EDA enhances scalability by enabling horizontal and vertical scaling. Horizontal scaling involves adding new components to the application without affecting existing ones. Vertical scaling allows for increasing the capacity of individual components independently. This decoupling of components facilitates scalability, allowing applications to handle growing loads or traffic without compromising performance.
Question 3: What are some common use cases for EDA in Golang applications?
Answer: EDA finds applications in various scenarios, including microservices architectures, where it enables communication and coordination among loosely coupled services. It is also useful in building event-driven pipelines for data processing and streaming applications. Additionally, EDA is leveraged in serverless architectures, where it facilitates event-based triggering of functions.
Question 4: What are the challenges associated with implementing EDA in Golang applications?
Answer: Implementing EDA introduces certain challenges. Managing event ordering and ensuring data consistency across distributed components can be complex. Additionally, handling failures and ensuring fault tolerance in distributed systems requires careful consideration. Furthermore, choosing appropriate event brokers and message formats, as well as designing effective event schemas, are important aspects to address.
Question 5: What are some best practices for designing EDA systems in Golang?
Answer: Best practices for EDA in Golang include defining clear and concise event schemas, utilizing appropriate message brokers, and implementing mechanisms for handling failures and retries. It is also essential to consider scalability and performance requirements when choosing event brokers and designing event schemas. Additionally, adopting a domain-driven design approach can help align event design with business concepts.
Question 6: What resources are available for learning more about EDA in Golang?
Answer: Numerous resources are available to deepen your understanding of EDA in Golang. The official Golang documentation provides comprehensive information on channels, message queues, and concurrency patterns. Additionally, there are books, online courses, and community forums dedicated to EDA and Golang. Hands-on experimentation and building small EDA applications can also be valuable learning experiences.
In summary, EDA offers significant benefits for Golang applications, including improved scalability, performance, maintainability, flexibility, and concurrency. By understanding the concepts and addressing the challenges associated with EDA, you can effectively leverage it to build robust and scalable Golang applications.
To delve deeper into EDA and Golang, we recommend exploring the resources mentioned above and experimenting with hands-on projects. This will further enhance your knowledge and enable you to harness the power of EDA in your Golang applications.
Exploring Event-Driven Architecture in Golang Applications
Event-driven architecture (EDA) is a software design pattern that emphasizes the use of events to trigger actions and facilitate communication between different parts of an application. In Golang, EDA can be implemented using channels, message queues, or event buses to pass messages between components.
One of the benefits of using EDA in Golang applications is that it can improve scalability and performance by decoupling the different components of the application. This allows each component to operate independently and at its own pace, without being affected by the performance of other components. Additionally, EDA can make it easier to develop and maintain complex applications by providing a clear and structured way to handle events.
Here are a few examples of how EDA can be used to improve the scalability, performance, and maintainability of Golang applications:
Example 1:
Consider a microservices architecture where each service is responsible for a specific task. Using EDA, each service can publish events to a message broker when it completes a task. Other services can then subscribe to these events and take appropriate actions.
Example 2:
In a data processing pipeline, EDA can be used to trigger the execution of different stages of the pipeline based on the occurrence of specific events. For example, when a new data file is added to a directory, an event can be published to trigger the first stage of the pipeline, which might involve parsing the data file.
Example 3:
In a serverless architecture, EDA can be used to trigger the execution of functions based on the occurrence of specific events. For example, when a new user signs up for a service, an event can be published to trigger a function that sends a welcome email to the user.
These are just a few examples of how EDA can be used to improve the scalability, performance, and maintainability of Golang applications. By understanding the basics of EDA and how to implement it in your own applications, you can take advantage of its benefits and build more efficient and effective software.
In summary, EDA is a powerful tool that can be used to improve the scalability, performance, and maintainability of Golang applications. By understanding the concepts and addressing the challenges associated with EDA, you can effectively leverage it to build robust and scalable Golang applications.
To delve deeper into EDA and Golang, we recommend exploring the resources mentioned above and experimenting with hands-on projects. This will further enhance your knowledge and enable you to harness the power of EDA in your Golang applications.
Conclusion
Event-driven architecture (EDA) offers a powerful approach to designing scalable, performant, and maintainable Golang applications. By embracing EDA principles and implementing them effectively, developers can unlock the full potential of their applications.
This exploration has highlighted the key concepts and benefits of EDA, including its ability to decouple application components, improve scalability and performance, and enhance maintainability. Through practical examples, we have demonstrated how EDA can be applied in various scenarios, from microservices architectures to data processing pipelines and serverless environments.
While EDA introduces certain challenges, such as managing event ordering and ensuring data consistency, these can be addressed through careful design and the adoption of best practices. By utilizing appropriate event brokers, defining clear event schemas, and implementing robust error handling mechanisms, developers can build resilient and fault-tolerant EDA systems.
As the software landscape continues to evolve, EDA will undoubtedly play an increasingly significant role. Its ability to facilitate asynchronous communication, support distributed systems, and enable reactive programming makes it an essential tool for building modern, responsive, and scalable Golang applications.
Youtube Video:
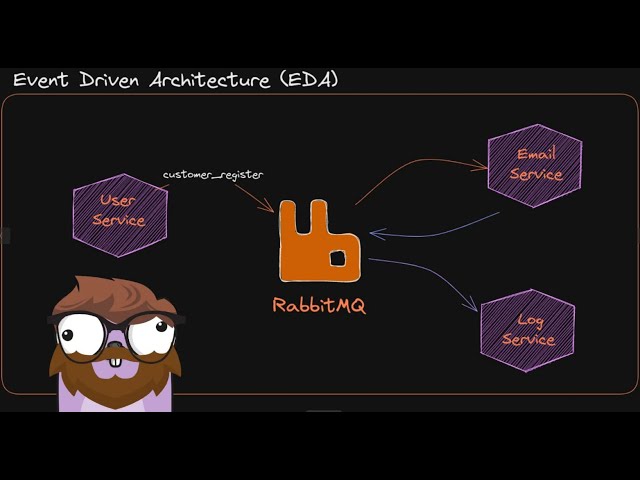