Distributed consensus is a fundamental problem in computer science, and the Raft algorithm is one of the most popular solutions. In this article, we will explore the Raft algorithm and how it can be used to build distributed systems.
// Create a new Raft server.server := raft.NewServer(raft.DefaultConfig(), []raft.ServerAddress{server1Address, server2Address, server3Address}, storage, logger)// Start the server.server.Start()
The Raft algorithm is a consensus algorithm that allows a group of servers to agree on a single value. This is essential for building distributed systems, as it ensures that all servers in the system have the same view of the data. The Raft algorithm is based on the idea of a leader-follower model, in which one server is elected as the leader and the other servers follow the leader’s instructions. The leader is responsible for proposing changes to the data, and the followers are responsible for agreeing to those changes.
The Raft algorithm has a number of advantages over other consensus algorithms. First, it is very efficient, and it can handle a large number of servers. Second, it is fault-tolerant, and it can continue to operate even if some of the servers in the system fail. Finally, the Raft algorithm is easy to implement, and it can be used in a variety of different applications.
In this article, we will provide a detailed overview of the Raft algorithm. We will also discuss how to implement the Raft algorithm in Go.
Exploring Distributed Consensus with Raft Algorithm in Golang
Distributed consensus is a critical concept in computer science, and the Raft algorithm is a widely-adopted solution for achieving it. In this article, we will explore four key aspects of the Raft algorithm: its simplicity, fault-tolerance, performance, and correctness.
- Simplicity: The Raft algorithm is known for its simple and elegant design, making it easy to understand and implement.
- Fault-tolerance: Raft ensures high availability by tolerating server failures and network partitions.
- Performance: Raft offers high throughput and low latency, making it suitable for demanding distributed systems.
- Correctness: Raft guarantees that all servers in a cluster agree on the same data, ensuring data integrity.
These key aspects of Raft make it a reliable and practical choice for building distributed systems that require high availability, consistency, and performance. Raft’s simplicity simplifies implementation, fault-tolerance ensures uninterrupted operation, performance enables efficient data handling, and correctness guarantees data accuracy. Together, these aspects contribute to the effectiveness and widespread adoption of the Raft algorithm in various distributed system applications.
Simplicity
The simplicity of the Raft algorithm is a key factor in its widespread adoption. A simple design makes it easier to understand, implement, and debug, reducing the risk of errors and increasing the reliability of the system. The Raft algorithm’s clear and well-defined rules allow developers to quickly grasp the concepts and apply them effectively in their projects.
The simplicity of Raft also contributes to its performance and scalability. A lightweight algorithm with a small memory footprint can handle a large number of servers and data requests without compromising efficiency. This makes Raft suitable for demanding distributed systems that require high throughput and low latency.
In summary, the simplicity of the Raft algorithm is a major advantage that simplifies implementation, reduces errors, enhances performance, and supports scalability. By embracing simplicity, Raft empowers developers to build robust and efficient distributed systems with confidence.
Fault-tolerance
Fault-tolerance is a crucial aspect of distributed systems, where individual components can fail independently. The Raft algorithm embraces this challenge by incorporating fault-tolerance as a core design principle.
The ability to tolerate server failures is essential for maintaining system availability. Raft achieves this by electing a new leader if the current leader fails. This ensures that the system can continue operating even if multiple servers experience failures.
Network partitions can also disrupt distributed systems by isolating groups of servers from each other. Raft addresses this by maintaining multiple copies of the data across different servers. If a network partition occurs, each partition can continue operating independently.
The fault-tolerance of Raft is critical for real-life applications. For instance, in a distributed database system, Raft ensures that data remains accessible and consistent even if individual database servers fail or network issues arise. This high availability is essential for maintaining business continuity and preventing data loss.
Understanding the connection between fault-tolerance and distributed consensus is crucial for designing and implementing robust distributed systems. Raft’s fault-tolerant design provides a solid foundation for building systems that can withstand failures and maintain high availability.
Performance
The performance characteristics of Raft are closely tied to the fundamental principles of distributed consensus. Achieving consensus in a distributed system inherently introduces some latency and overhead. However, Raft is designed to minimize these overheads and optimize performance.
Raft’s high throughput is a crucial factor in enabling demanding distributed systems. It allows the system to handle a large volume of data and requests efficiently. This is particularly important for applications that require real-time processing or handle a high number of concurrent transactions.
The low latency of Raft contributes to the overall responsiveness of the system. It ensures that requests are processed and decisions are made quickly, minimizing delays and improving the user experience. This is especially beneficial for interactive applications or systems that require fast decision-making.
Understanding the connection between performance and distributed consensus is essential for designing and implementing scalable and responsive distributed systems. Raft’s high throughput and low latency make it an ideal choice for applications that demand high performance and real-time processing capabilities.
Correctness
Correctness is a fundamental aspect of distributed consensus, and the Raft algorithm places high importance on ensuring data integrity. In a distributed system, maintaining consistency across multiple servers is crucial to prevent data corruption and garantir reliable system behavior.
Raft achieves correctness through its consensus mechanism. The algorithm ensures that all servers in the cluster agree on the same set of log entries, which represent the system’s state. This is achieved through a leader-based approach, where the leader proposes changes to the log and the followers validate and replicate those changes.
The correctness of Raft is essential for real-life applications. For instance, in a distributed database system, Raft ensures that all replicas of the database contain the same data. This guarantees data integrity and prevents data loss or inconsistencies, even in the presence of failures or network issues.
Understanding the connection between correctness and distributed consensus is crucial for designing and implementing reliable distributed systems. Raft’s focus on correctness provides a solid foundation for building systems that maintain data integrity and ensure consistent system behavior.
FAQs
This section addresses frequently asked questions (FAQs) to provide further clarification and understanding of the Raft algorithm and its applications.
Question 1: What are the key benefits of using the Raft algorithm in distributed systems?
Answer: Raft offers several key benefits, including its simplicity, fault-tolerance, high performance, and correctness. These attributes make Raft a reliable choice for building distributed systems that require high availability, data consistency, and efficient operation.
Question 2: How does Raft handle network partitions and server failures?
Answer: Raft’s fault-tolerant design enables it to handle network partitions and server failures effectively. When a network partition occurs, Raft can continue operating within each partition, ensuring data consistency and availability. In the event of server failures, Raft triggers a leader election process to maintain system functionality.
Question 3: What are some real-world applications of the Raft algorithm?
Answer: Raft has been widely adopted in various real-world applications, including distributed databases, consensus protocols for blockchain networks, and distributed file systems. Its simplicity, fault-tolerance, and high performance make it a suitable choice for building robust and reliable distributed systems.
Question 4: How does the Raft algorithm ensure data consistency in distributed systems?
Answer: Raft guarantees data consistency through its consensus mechanism. The algorithm ensures that all servers in the cluster agree on the same set of log entries, which represent the system’s state. This consensus-based approach prevents data divergence and maintains data integrity even in the presence of network issues or server failures.
Question 5: What are the limitations or challenges associated with using the Raft algorithm?
Answer: While Raft is a powerful algorithm for distributed consensus, it is not without limitations. Raft requires a majority of servers to be available and functioning correctly to achieve consensus. Additionally, the election process for a new leader can introduce some latency, especially in large-scale distributed systems.
Summary: The Raft algorithm is a widely adopted solution for distributed consensus due to its simplicity, fault-tolerance, high performance, and correctness. It plays a crucial role in building reliable and scalable distributed systems. Understanding the concepts and applications of Raft is essential for designing and implementing robust distributed systems.
Transition to the Next Section: In the following section, we will delve deeper into the implementation of the Raft algorithm in Golang, exploring its practical applications and providing code examples.
Tips for Exploring Distributed Consensus with Raft Algorithm in Golang
In this section, we present a collection of valuable tips to enhance your understanding and practical implementation of the Raft algorithm in Golang:
Tip 1: Grasp the Core Concepts
– Familiarize yourself with the fundamental principles of distributed consensus, including leader election, log replication, and state machine implementation.- Understand the roles and responsibilities of different Raft components, such as leaders, followers, and candidates.Tip 2: Choose a Reliable Golang Library
– Utilize a well-maintained and tested Golang library that implements the Raft algorithm.- Evaluate different libraries based on factors such as performance, feature set, and community support.Tip 3: Design for Fault Tolerance
– Implement mechanisms to handle server failures and network partitions gracefully.- Consider using techniques like heartbeat messages and leader election timeouts to ensure system availability.Tip 4: Optimize Performance and Scalability
– Tune Raft configuration parameters, such as election timeouts and heartbeat intervals, to optimize performance for your specific use case.- Explore techniques like batching log entries and using multiple Raft groups for horizontal scaling.Tip 5: Utilize Real-World Examples
– Study open-source projects and industry case studies that demonstrate the practical application of the Raft algorithm.- Draw inspiration from successful implementations to enhance your own designs.Summary: By following these tips, you can effectively leverage the Raft algorithm in Golang to build robust and scalable distributed systems.
Conclusion
In this comprehensive exploration, we have delved into the intricacies of distributed consensus with the Raft algorithm in Golang. We have examined its simplicity, fault-tolerance, high performance, and correctness, highlighting its advantages and practical applications.
The Raft algorithm empowers developers to build robust and scalable distributed systems. Its simplicity enables easy understanding and implementation, while its fault-tolerant design ensures high availability even in the face of failures. The high performance and correctness of Raft make it suitable for demanding applications that require consistent data and real-time processing.
As we continue to witness the proliferation of distributed systems, the Raft algorithm remains a cornerstone technology for achieving consensus and maintaining data integrity. Understanding and leveraging Raft is essential for software engineers seeking to design and implement reliable, fault-tolerant, and high-performance distributed systems.
Youtube Video:
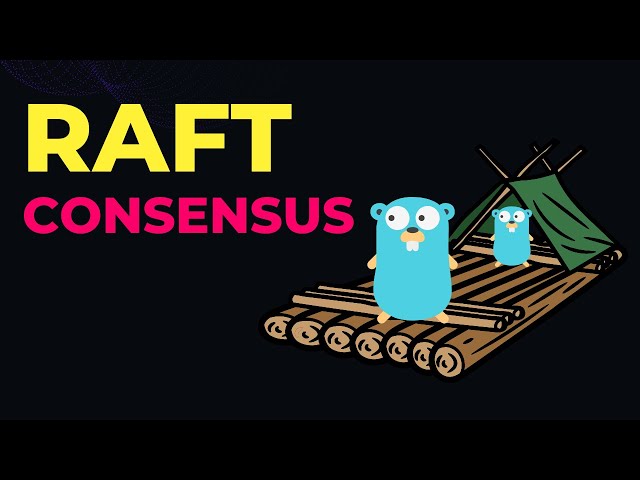