Design patterns are reusable solutions to commonly occurring problems in software development. They provide a blueprint for structuring code, making it easier to read, understand, and maintain. Go, a programming language known for its simplicity, concurrency, and efficiency, offers a rich set of design patterns that can help developers create robust and scalable applications.
// Singleton pattern ensures that a class has only one instance and provides a global point of access to that instance. type Singleton struct{} var instance Singleton func GetInstance() Singleton { if instance == nil { instance = &Singleton{} } return instance }
Design patterns play a crucial role in software development by promoting code reusability, improving code readability, and ensuring maintainability. Historically, design patterns emerged as a way to capture and document best practices in software engineering, providing a common vocabulary and set of solutions for developers to leverage.
This article will delve into the world of design patterns in Go, exploring their benefits, real-world applications, and best practices for their implementation. We will cover fundamental patterns like Singleton, Factory, and Strategy, demonstrating their usage with practical examples and highlighting their impact on software quality.
Exploring Design Patterns in Golang
Design patterns are a key aspect of software development, providing reusable solutions to commonly occurring problems. In Go, design patterns help create robust and scalable applications.
- Code Reusability: Design patterns allow developers to reuse proven solutions, saving time and effort.
- Improved Code Readability: By following established patterns, code becomes more readable and easier to understand.
- Maintainability: Design patterns promote code maintainability by organizing code into well-defined structures.
For example, the Singleton pattern ensures that a class has only one instance, providing a global point of access to that instance. This pattern is useful in scenarios where a single, shared resource is required, such as a database connection pool or a logging service. By leveraging design patterns like Singleton, developers can write code that is more efficient, reliable, and easier to maintain.
Code Reusability
Design patterns foster code reusability by providing tried-and-tested solutions to common software development challenges. By leveraging these patterns, developers can avoid reinventing the wheel, significantly reducing development time and effort. Furthermore, code reuse promotes consistency and reduces the risk of introducing errors, as developers can rely on well-established and documented solutions.
For instance, the Singleton pattern ensures that a class has only one instance, providing a global point of access to that instance. This pattern is particularly useful when dealing with resources that should only have a single instance, such as database connections or logging services. By utilizing the Singleton pattern, developers can ensure that these resources are managed efficiently and consistently throughout the application.
In summary, design patterns promote code reusability, reducing development time and effort while enhancing code quality and maintainability. Embracing design patterns empowers developers to create robust and scalable software applications effectively.
Improved Code Readability
In the context of “Exploring Design Patterns in Golang: Best Practices for Software Development,” improved code readability plays a vital role in enhancing the overall quality and maintainability of software applications.
- Consistent Coding Style: Design patterns enforce consistent coding styles and conventions, making it easier for developers to read and comprehend code written by others.
- Reduced Cognitive Load: By following established patterns, developers can reduce their cognitive load, allowing them to focus on the specific logic and functionality of the code rather than spending time deciphering complex or unfamiliar coding styles.
- Enhanced Collaboration: Improved code readability fosters better collaboration among team members, as developers can more easily understand and contribute to each other’s work, leading to increased productivity and code quality.
- Effective Code Reviews: Design patterns facilitate more effective code reviews, as reviewers can quickly grasp the intent and structure of the code, enabling them to provide valuable feedback and identify potential issues.
In summary, improved code readability, a key aspect of design patterns in Go, contributes to the creation of software that is not only functional but also maintainable, understandable, and collaborative, ultimately leading to higher quality software development.
Maintainability
Maintainability is a crucial aspect of software development, ensuring that code can be easily modified, extended, and debugged throughout its lifecycle. Design patterns play a significant role in promoting code maintainability by providing well-defined and structured solutions to common software development problems.
By organizing code into well-defined structures, design patterns help developers create modular and flexible codebases. This modularity allows developers to make changes to specific parts of the code without affecting the rest of the system. As a result, code becomes more manageable and easier to maintain, reducing the risk of introducing bugs and errors.
For example, the Factory pattern provides a way to create objects without specifying the exact class of the object to be created. This allows developers to easily add new types of objects to the system without modifying the existing code. The Strategy pattern, on the other hand, allows developers to define a family of algorithms and encapsulate each algorithm in a separate class. This makes it easy to switch between different algorithms without modifying the client code.
In summary, design patterns contribute to the maintainability of software applications by providing structured and modular codebases. This makes it easier for developers to make changes, add new features, and fix bugs, ultimately leading to more robust and reliable software systems.
FAQs on Exploring Design Patterns in Golang
This section addresses frequently asked questions (FAQs) regarding the exploration of design patterns in Go, providing concise answers to common concerns and misconceptions.
Question 1: What are the key benefits of utilizing design patterns in Go?
Answer: Design patterns offer numerous benefits, including enhanced code reusability, improved code readability, and increased code maintainability. They promote consistency, reduce development time and effort, and facilitate effective collaboration among development teams.
Question 2: Are design patterns essential for every Go project?
Answer: While design patterns provide valuable guidance, they are not mandatory for every Go project. However, understanding and applying design patterns when appropriate can significantly improve the quality, maintainability, and scalability of software applications.
Question 3: How do design patterns relate to object-oriented programming (OOP) in Go?
Answer: Design patterns complement OOP principles in Go. They provide a structured approach to organizing and designing code, building upon the concepts of encapsulation, inheritance, and polymorphism. Design patterns help developers create flexible and extensible software architectures.
Question 4: What are some common design patterns used in Go?
Answer: Go supports a wide range of design patterns, including Creational patterns (e.g., Factory, Builder, Singleton), Structural patterns (e.g., Adapter, Decorator, Facade), and Behavioral patterns (e.g., Strategy, Observer, Command). The choice of design patterns depends on the specific requirements of the software application.
Question 5: How can I learn more about design patterns in Go?
Answer: There are numerous resources available to learn about design patterns in Go, including books, online courses, tutorials, and community forums. Exploring these resources and practicing the application of design patterns in real-world projects can enhance your understanding and proficiency.
In summary, design patterns offer a valuable toolkit for Go developers to create robust, maintainable, and extensible software applications. By addressing common concerns and providing practical guidance, this FAQ section aims to empower developers in their exploration of design patterns and their effective application in Go projects.
Transitioning to the next article section: This article further delves into the practical implementation of design patterns in Go, providing real-world examples and best practices to guide developers in leveraging these patterns effectively.
Tips for Exploring Design Patterns in Go
Design patterns provide a proven approach to solving common software development challenges. By leveraging design patterns in Go, developers can create robust, maintainable, and scalable applications. Here are a few tips to help you get started:
Tip 1: Understand the Problem Domain
Before applying design patterns, it’s crucial to thoroughly understand the problem domain and the specific challenges you’re trying to solve. This will help you select the most appropriate design patterns for your needs.
Tip 2: Choose the Right Patterns
Go offers a wide range of design patterns, each with its own strengths and use cases. Carefully consider the requirements of your application and choose the patterns that best fit your needs. Avoid using patterns indiscriminately.
Tip 3: Follow Best Practices
When implementing design patterns, follow established best practices to ensure their effectiveness and maintainability. This includes adhering to naming conventions, using dependency inversion, and avoiding unnecessary complexity.
Tip 4: Refactor and Adapt
Design patterns are not static solutions but rather guidelines that can be adapted to specific scenarios. Don’t hesitate to refactor and adjust patterns as needed to fit the unique requirements of your application.
Tip 5: Seek Continuous Improvement
Stay up-to-date with the latest developments in design patterns and best practices. Attend conferences, read books, and engage in online discussions to enhance your knowledge and skills.
By following these tips, you can effectively explore and apply design patterns in your Go projects, leading to high-quality, maintainable, and scalable software solutions.
Conclusion
In this article, we have explored the significance of design patterns in Go and their impact on software development best practices. We have discussed the benefits of code reusability, improved code readability, and enhanced code maintainability that design patterns provide.
By understanding and applying design patterns effectively, Go developers can create robust, maintainable, and scalable software applications. We encourage you to delve deeper into the world of design patterns, experiment with different patterns, and continuously seek improvement to elevate your software development skills.
The exploration of design patterns is an ongoing journey, and we believe that the knowledge and techniques covered in this article will serve as a valuable foundation for your future endeavors. Embrace the power of design patterns and unlock the full potential of Go in your software development projects.
Youtube Video:
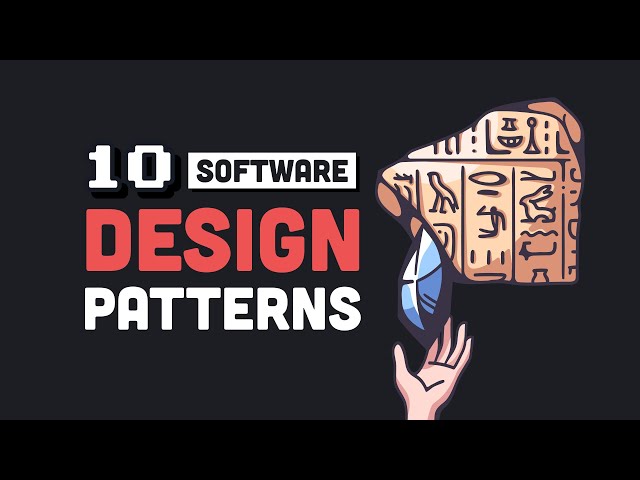