At the core of computer science lies the study of data structures and algorithms, which provide a foundation for organizing, storing, and manipulating data efficiently. “Exploring Data Structures and Algorithms in Golang: Core Concepts” delves into these fundamental concepts, using the versatile and performant Go programming language.
This comprehensive guide takes a practical approach, introducing essential data structures such as arrays, linked lists, stacks, and queues, explaining their strengths and applications. It also covers fundamental algorithms for searching, sorting, and manipulating data, empowering readers to develop efficient and scalable solutions.
// Example of a linked list in Golangtype node struct { data int next *node}func main() { head := &node{data: 1} head.next = &node{data: 2} head.next.next = &node{data: 3} // Iterate over the linked list for current := head; current != nil; current = current.next { fmt.Println(current.data) }}
The book’s focus on Go, a modern and widely adopted language known for its speed and concurrency, makes it particularly valuable for developers seeking to leverage these capabilities in their projects. By understanding the core concepts presented in “Exploring Data Structures and Algorithms in Golang: Core Concepts,” readers gain a solid foundation for building robust and efficient software solutions.
Exploring Data Structures and Algorithms in Golang
Data structures and algorithms lie at the heart of computer science, providing the foundation for organizing, storing, and manipulating data efficiently. Exploring these concepts in Golang, a modern and performant programming language, empowers developers to build robust and scalable software solutions.
- Core Data Structures: Arrays, linked lists, stacks, and queues form the cornerstone of data storage and manipulation, each with unique characteristics and applications.
- Essential Algorithms: Searching, sorting, and manipulating algorithms provide efficient techniques for finding, organizing, and transforming data, optimizing performance and reducing complexity.
Understanding these key aspects enables developers to make informed decisions about data structures and algorithms, matching them to specific requirements. For instance, a linked list excels in scenarios requiring frequent insertions and deletions, while a binary search tree optimizes searching operations. By leveraging the strengths of each data structure and algorithm, developers can design efficient and scalable solutions for real-world problems.
Core Data Structures
In “Exploring Data Structures and Algorithms in Golang: Core Concepts,” a key focus lies in understanding the fundamental data structures that serve as building blocks for efficient data management. Arrays, linked lists, stacks, and queues each possess distinct properties and use cases, making them essential components in a programmer’s toolkit.
Arrays, with their contiguous memory allocation, provide efficient access and manipulation of elements. Linked lists, on the other hand, excel in scenarios where frequent insertions and deletions are required, as they allow for dynamic memory allocation and efficient handling of node references.
Stacks and queues follow the last-in, first-out (LIFO) and first-in, first-out (FIFO) principles, respectively. Stacks are particularly useful in scenarios involving function calls and recursion, while queues find applications in message handling and task scheduling.
By comprehending the strengths and weaknesses of these core data structures, developers gain the ability to select the most appropriate one for their specific requirements. This understanding empowers them to design efficient and scalable solutions, optimizing performance and ensuring data integrity.
In summary, exploring core data structures forms the foundation of “Exploring Data Structures and Algorithms in Golang: Core Concepts,” providing a comprehensive understanding of the fundamental building blocks for efficient data storage and manipulation in Golang.
Essential Algorithms
Essential algorithms play a pivotal role in “Exploring Data Structures and Algorithms in Golang: Core Concepts,” as they provide the means to efficiently manipulate and transform data, optimizing performance and enhancing the overall functionality of software systems.
Searching algorithms, such as linear search and binary search, enable efficient retrieval of data from data structures. Sorting algorithms, including bubble sort, insertion sort, and merge sort, organize data in a specific order, facilitating efficient retrieval and processing. Manipulating algorithms, such as reversing a linked list or finding the maximum element in an array, provide essential operations for data transformation and analysis.
The connection between essential algorithms and “Exploring Data Structures and Algorithms in Golang: Core Concepts” lies in the fact that these algorithms are intimately tied to the efficient implementation and utilization of data structures. By understanding the capabilities and limitations of different algorithms, developers can make informed decisions about which ones to employ for specific tasks, ensuring optimal performance and resource utilization.
For instance, if a developer needs to efficiently search for an element in a large dataset, they might opt for a binary search algorithm, which has a time complexity of O(log n), compared to a linear search with a time complexity of O(n). Similarly, when sorting a large dataset, a merge sort or quick sort algorithm would be more efficient than a bubble sort, especially for datasets with a large number of elements.
In summary, essential algorithms are an integral part of “Exploring Data Structures and Algorithms in Golang: Core Concepts” as they provide the means to efficiently manipulate and transform data, optimize performance, and enhance the overall functionality of software systems.
Frequently Asked Questions about “Exploring Data Structures and Algorithms in Golang
This section addresses common questions and misconceptions surrounding the book “Exploring Data Structures and Algorithms in Golang: Core Concepts,” providing clear and concise answers to guide your understanding.
Question 1: Is this book suitable for beginners with no prior knowledge of data structures and algorithms?
Yes, this book is designed to be accessible to beginners, assuming no prior knowledge of data structures and algorithms. It starts with the basics and gradually introduces more advanced concepts, providing a solid foundation for understanding these fundamental computer science topics.
Question 2: What are the key benefits of using Golang for data structures and algorithms?
Golang, known for its simplicity, efficiency, and concurrency features, offers several advantages for implementing data structures and algorithms. Its built-in support for concurrency allows for efficient handling of multiple tasks simultaneously, enhancing the performance of algorithms that can be parallelized.
Question 3: Does this book cover advanced data structures and algorithms, such as trees and graphs?
While “Exploring Data Structures and Algorithms in Golang: Core Concepts” primarily focuses on fundamental data structures and algorithms, it does introduce some advanced concepts, including binary trees and basic graph algorithms. For a more comprehensive exploration of advanced data structures and algorithms, you may want to refer to specialized resources.
Question 4: How can I apply the concepts learned from this book to real-world projects?
Understanding data structures and algorithms is essential for developing efficient and scalable software solutions. By applying the concepts presented in this book, you can design and implement data-driven applications that can handle complex data efficiently, improving the performance and reliability of your software systems.
Question 5: Are there any prerequisites before reading this book?
A basic understanding of the Golang programming language is recommended before delving into this book. Familiarity with basic programming concepts, such as variables, data types, and control flow, will enhance your comprehension of the material presented.
Question 6: What is the target audience for this book?
This book is primarily intended for software developers, computer science students, and anyone interested in gaining a solid foundation in data structures and algorithms using the Golang programming language. It is suitable for both beginners with no prior knowledge and those seeking to refresh their understanding of these fundamental concepts.
In summary, “Exploring Data Structures and Algorithms in Golang: Core Concepts” provides a comprehensive and accessible introduction to data structures and algorithms, utilizing the strengths of the Golang programming language. By addressing common questions and misconceptions, this FAQ section aims to clarify your understanding and guide your learning journey.
To further enhance your knowledge, consider exploring the book’s accompanying website for additional resources, exercises, and community discussions.
Exploring Data Structures and Algorithms in Golang
In “Exploring Data Structures and Algorithms in Golang: Core Concepts,” the focus lies on providing a comprehensive understanding of fundamental data structures and algorithms, using the versatile and performant Go programming language. This book empowers readers to build robust and scalable software solutions by mastering these essential concepts.
At the heart of the book lies the exploration of core data structures, including arrays, linked lists, stacks, and queues. Each data structure possesses unique characteristics and use cases, catering to specific data storage and manipulation requirements. The book delves into the strengths and weaknesses of these structures, enabling readers to make informed decisions about their selection.
Furthermore, the book emphasizes essential algorithms, such as searching, sorting, and manipulating algorithms, which play a crucial role in organizing, retrieving, and transforming data efficiently. These algorithms provide a systematic approach to solving common programming challenges, optimizing performance, and enhancing the overall functionality of software systems.
By understanding the interplay between data structures and algorithms, readers gain the ability to design and implement efficient solutions for real-world problems. The book presents numerous examples and exercises, reinforcing the concepts and guiding readers through practical applications.
In summary, “Exploring Data Structures and Algorithms in Golang: Core Concepts” serves as a valuable resource for software developers, computer science students, and anyone seeking to master the fundamentals of data structures and algorithms using Golang. Its comprehensive coverage, practical approach, and focus on core concepts empower readers to build robust and scalable software solutions.
Conclusion
In exploring the depths of “Exploring Data Structures and Algorithms in Golang: Core Concepts,” we have embarked on a journey that unravels the fundamental principles of data organization and manipulation in computer science. Through a comprehensive examination of core data structures and essential algorithms, this book empowers us to construct robust and scalable software solutions.
This exploration has illuminated the significance of data structures, such as arrays, linked lists, stacks, and queues, and their diverse applications in data storage and retrieval. We have gained insights into the strengths and limitations of each structure, enabling us to make informed decisions when selecting the most appropriate one for specific requirements.
Furthermore, we have delved into the realm of essential algorithms, including searching, sorting, and manipulating algorithms, which provide systematic approaches to solving common programming challenges. By mastering these algorithms, we can optimize performance, enhance efficiency, and elevate the functionality of our software systems.
As we conclude our exploration of “Exploring Data Structures and Algorithms in Golang: Core Concepts,” let us carry forward the knowledge we have acquired and apply it to the design and implementation of efficient and scalable software solutions. May this journey inspire us to continuously explore the depths of computer science and contribute to the advancement of technology.
Youtube Video:
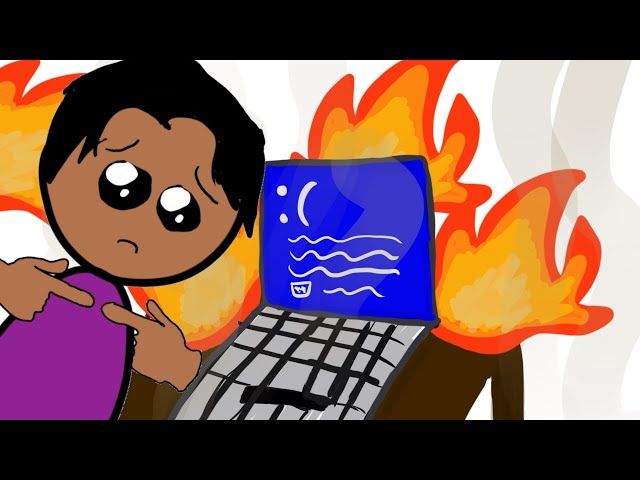